基于#include <stdio.h> int fcount(char str[],char s); int main(void) { char str[80],s; gets(str); s=getchar(); printf("%d",fcount(str,s)); return 0; } /*考生在以下空白处定义函数*/ /*考生在以上空白处定义函数*/输入一个字符串,统计字符串中指定字符出现次数并输出。【输入格式】 在第一行中输入不超过80个字符长度的、以回车结束的非空字符串:在第二行中输入1个指定字符。【输出格式】 在一行中输出字符串中指定字符的出现次数。【输入样例】 Hello World!1 【输出样例】3 【函数定义要求 请在该程序文件中,实现函数int fcount(charstr1.chars)统计字符串str中字符s的出现次数,并作为函数的返回结果。
时间: 2024-03-11 13:50:58 浏览: 79
好的,这是相应的代码:
```c
#include <stdio.h>
int fcount(char str[], char s) {
int count = 0;
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] == s) {
count++;
}
}
return count;
}
int main(void) {
char str[80], s;
gets(str);
s = getchar();
printf("%d", fcount(str, s));
return 0;
}
```
在这个程序中,我们首先定义了一个函数 `fcount`,它有两个参数,分别是指向字符数组的指针 `str` 和字符 `s`。函数的作用是统计字符串 `str` 中指定字符 `s` 出现的次数,并返回这个次数。
在主程序中,我们首先定义了一个字符数组 `str` 和一个字符变量 `s`。然后从标准输入中读入一个字符串 `str` 和一个字符 `s`。接着调用 `fcount` 函数,将统计结果打印出来。
注意,在 `gets` 函数中,我们没有设定最大字符数,因为题目规定字符串长度不超过 80。另外,在 `fcount` 函数中,我们使用了 C 语言中的字符串结束符 `'\0'` 来判断字符串是否结束。
相关问题
基于#include <stdio.h> int fcount(char str[],char s); int main(void) { char str[80],s; gets(str); s=getchar(); printf("%d",fcount(str,s)); return 0; }输入一个字符串,统计字符串中指定字符出现次数并输出。【输入格式】 在第一行中输入不超过80个字符长度的、以回车结束的非空字符串:在第二行中输入1个指定字符。【输出格式】 在一行中输出字符串中指定字符的出现次数。【输入样例】 Hello World!1 【输出样例】3 【函数定义要求 请在该程序文件中,实现函数int fcount(charstr1.chars)统计字符串str中字符s的出现次数,并作为函数的返回结果。
以下是函数的实现:
```c++
#include <stdio.h>
int fcount(char str[], char s);
int main(void) {
char str[80], s;
gets(str);
s = getchar();
printf("%d", fcount(str, s));
return 0;
}
int fcount(char str[], char s) {
int count = 0;
int i = 0;
while (str[i] != '\0') {
if (str[i] == s) {
count++;
}
i++;
}
return count;
}
```
主要思路是遍历字符串中的每一个字符,如果等于指定字符,就将计数器加一。最后返回计数器的值即可。需要注意的是,使用 `gets` 函数存在安全问题,应该使用更安全的函数(如 `fgets`)进行字符串输入。此外,在输入指定字符时,需要注意输入的字符可能包含回车符,因此需要使用 `getchar` 函数进行输入。
#include <stdio.h>#include <string.h>void mystrcat(char *str1, char *str2) { strcat(str1, str2);}int main() { char str1[100], str2[100]; scanf("%s %s", str1, str2); mystrcat(str1, str2); printf("%s", str1); return 0;}添加注释
```
#include <stdio.h>
#include <string.h>
// 自定义字符串拼接函数,将 str2 拼接到 str1 的末尾
void mystrcat(char *str1, char *str2) {
strcat(str1, str2);
}
int main() {
char str1[100], str2[100];
scanf("%s %s", str1, str2); // 从控制台输入两个字符串
mystrcat(str1, str2); // 调用自定义函数拼接字符串
printf("%s", str1); // 输出拼接后的字符串
return 0;
}
```
该程序是一个字符串拼接程序,通过自定义函数 mystrcat() 实现字符串拼接功能。该函数调用了标准库函数 strcat(),将第二个字符串拼接到第一个字符串的末尾。在主函数中,通过 scanf() 从控制台输入两个字符串,然后调用自定义函数 mystrcat() 拼接字符串,最后输出拼接后的字符串。
阅读全文
相关推荐
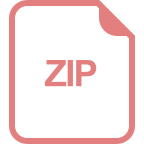
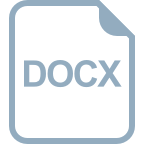
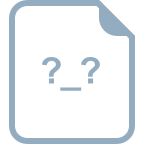














