python写一个旅游管理系统,写出景点,交通方式,电话,邮箱,景点推荐
时间: 2023-11-21 14:52:19 浏览: 150
好的,下面是一个简单的旅游管理系统示例,包括景点、交通方式、联系方式和景点推荐。注释已经在代码中添加:
```python
class Attraction:
# 定义景点类
def __init__(self, name, description):
self.name = name
self.description = description
def __str__(self):
return f"{self.name}: {self.description}"
class Transportation:
# 定义交通方式类
def __init__(self, name, description):
self.name = name
self.description = description
def __str__(self):
return f"{self.name}: {self.description}"
class Contact:
# 定义联系方式类
def __init__(self, phone, email):
self.phone = phone
self.email = email
def __str__(self):
return f"Phone: {self.phone}\nEmail: {self.email}"
class TouristAttraction:
# 定义旅游景点类
def __init__(self, name, description, transportation, contact):
self.name = name
self.description = description
self.transportation = transportation
self.contact = contact
def __str__(self):
return f"{self.name}\n{self.description}\n\nTransportation:\n{self.transportation}\n\nContact:\n{self.contact}"
class TouristAttractionRecommendation:
# 定义旅游景点推荐类
def __init__(self, attractions):
self.attractions = attractions
def recommend(self):
return self.attractions[0]
if __name__ == "__main__":
# 创建景点
tiananmen = Attraction("Tiananmen Square", "The heart of Beijing")
great_wall = Attraction("Great Wall of China", "The longest wall in the world")
# 创建交通方式
taxi = Transportation("Taxi", "Convenient but expensive")
subway = Transportation("Subway", "Cheap but crowded")
# 创建联系方式
tiananmen_contact = Contact("010-6559-0000", "tiananmen@example.com")
great_wall_contact = Contact("010-1234-5678", "greatwall@example.com")
# 创建旅游景点
tiananmen_square = TouristAttraction("Tiananmen Square", "The heart of Beijing", subway, tiananmen_contact)
great_wall_of_china = TouristAttraction("Great Wall of China", "The longest wall in the world", taxi, great_wall_contact)
# 创建旅游景点推荐
attractions = [tiananmen_square, great_wall_of_china]
recommendation = TouristAttractionRecommendation(attractions)
print("Recommended attraction: ", recommendation.recommend())
```
以上代码是一个基本的旅游管理系统示例,可以根据需要进行扩展和优化。
阅读全文
相关推荐
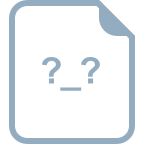

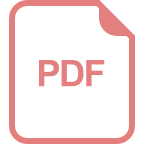
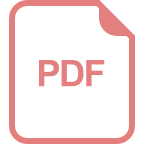
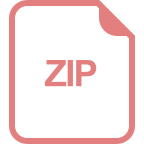
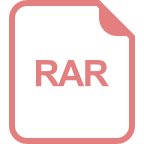
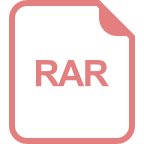
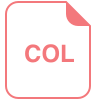
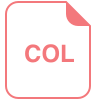



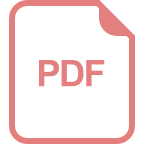
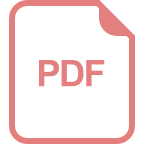
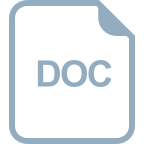