自助点餐系统c++
时间: 2023-08-03 10:16:42 浏览: 120
下面是一个简单的基于C++实现的自助点餐系统代码示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <iomanip>
#include <string>
using namespace std;
// 菜品结构体
struct Dish {
string name; // 菜名
double price; // 价格
string desc; // 描述
};
// 读取菜单信息
vector<Dish> readMenu() {
vector<Dish> menu;
ifstream ifs("menu.txt");
if (!ifs.is_open()) {
cout << "菜单文件不存在!" << endl;
return menu;
}
Dish dish;
while (ifs >> dish.name >> dish.price) {
getline(ifs, dish.desc);
menu.push_back(dish);
}
ifs.close();
return menu;
}
// 展示菜单
void showMenu(const vector<Dish>& menu) {
cout << "****************** 菜单 ******************" << endl;
cout << setw(10) << "编号" << setw(10) << "名称" << setw(10) << "价格" << setw(20) << "描述" << endl;
for (int i = 0; i < menu.size(); i++) {
cout << setw(10) << i + 1 << setw(10) << menu[i].name << setw(10) << menu[i].price << setw(20) << menu[i].desc << endl;
}
cout << "*******************************************" << endl;
}
// 点菜
void order(vector<Dish>& menu, vector<int>& orderList) {
int dishId, num;
cout << "请输入菜品编号和数量(输入0结束):" << endl;
while (true) {
cin >> dishId;
if (dishId == 0) {
break;
}
cin >> num;
orderList[dishId - 1] += num;
}
// 输出当前点餐情况
cout << "************ 当前点餐情况 ************" << endl;
cout << setw(10) << "编号" << setw(10) << "名称" << setw(10) << "数量" << setw(10) << "小计" << endl;
double total = 0;
for (int i = 0; i < menu.size(); i++) {
if (orderList[i] > 0) {
double subtotal = orderList[i] * menu[i].price;
total += subtotal;
cout << setw(10) << i + 1 << setw(10) << menu[i].name << setw(10) << orderList[i] << setw(10) << subtotal << endl;
}
}
cout << "总计:" << total << endl;
cout << "***************************************" << endl;
}
// 结账
void checkout(vector<Dish>& menu, const vector<int>& orderList) {
double total = 0;
for (int i = 0; i < menu.size(); i++) {
if (orderList[i] > 0) {
double subtotal = orderList[i] * menu[i].price;
total += subtotal;
}
}
cout << "总计:" << total << endl;
cout << "请输入支付金额:" << endl;
double payment;
cin >> payment;
if (payment < total) {
cout << "支付金额不足,请重新输入:" << endl;
cin >> payment;
}
double change = payment - total;
cout << "找零:" << change << endl;
// 保存订单信息到文件
ofstream ofs("orders.txt", ios::app);
if (ofs.is_open()) {
ofs << "订单号:" << time(NULL) << endl;
for (int i = 0; i < menu.size(); i++) {
if (orderList[i] > 0) {
ofs << menu[i].name << " * " << orderList[i] << " = " << orderList[i] * menu[i].price << endl;
}
}
ofs << "总计:" << total << endl;
ofs << "支付:" << payment << endl;
ofs << "找零:" << change << endl;
ofs << "*******************************************" << endl;
ofs.close();
}
}
// 查询订单
void queryOrders() {
ifstream ifs("orders.txt");
if (!ifs.is_open()) {
cout << "暂无订单信息!" << endl;
return;
}
string line;
while (getline(ifs, line)) {
cout << line << endl;
}
ifs.close();
}
int main() {
vector<Dish> menu = readMenu();
vector<int> orderList(menu.size(), 0);
while (true) {
cout << "请选择操作:1.查看菜单 2.点菜 3.结账 4.查询订单 5.退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
showMenu(menu);
break;
case 2:
order(menu, orderList);
break;
case 3:
checkout(menu, orderList);
break;
case 4:
queryOrders();
break;
case 5:
return 0;
default:
cout << "无效操作,请重新选择!" << endl;
break;
}
}
return 0;
}
```
该示例代码包含了菜单读取、菜单展示、点菜、结账、订单查询等功能。具体实现细节可以根据实际需求进行调整。
相关推荐
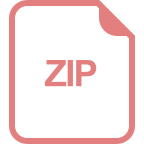
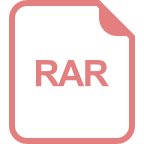














