python获取csv文件中的每个月的微博的点赞数趋势图并显示在网页上
时间: 2024-02-09 10:11:28 浏览: 78
要实现这个功能,需要使用Python的数据处理和可视化库,将CSV文件中的数据读取出来,并将每个月的点赞数数据分别存储到一个字典中,再将字典中的数据转换成趋势图显示在网页上。
以下是大致的实现步骤:
1. 使用Python的csv库读取CSV文件中的数据,并将每条微博的点赞数和时间信息存储到一个列表中。
2. 使用Python的datetime库将时间信息转换成月份,并将每个月的点赞数数据分别存储到一个字典中。
3. 使用Python的可视化库(如matplotlib)将字典中的数据转换成趋势图。
4. 使用Python的web框架(如Flask)创建一个网页,并将趋势图嵌入到网页中。
5. 在浏览器中访问网页,即可看到每个月的微博点赞数趋势图。
以下是一个简单的代码示例:
```python
import csv
import datetime
import matplotlib.pyplot as plt
from flask import Flask, render_template
app = Flask(__name__)
@app.route("/")
def index():
likes_dict = {}
with open('weibo_data.csv', 'r', encoding='utf-8') as f:
reader = csv.reader(f)
next(reader) # 跳过CSV文件的标题行
for row in reader:
dt = datetime.datetime.strptime(row[0], '%Y-%m-%d %H:%M:%S')
month = dt.strftime('%Y-%m') # 将时间信息转换成月份
if month not in likes_dict:
likes_dict[month] = []
likes_dict[month].append(int(row[1])) # 将点赞数数据存储到字典中
for month, likes in likes_dict.items():
plt.plot(likes, label=month) # 将每个月的点赞数数据转换成趋势图
plt.title('Likes Trend by Month')
plt.xlabel('Time')
plt.ylabel('Likes')
plt.legend()
plt.savefig('likes_trend.png') # 将趋势图保存为图片
return render_template('index.html', image_file='likes_trend.png') # 将趋势图显示在网页上
if __name__ == "__main__":
app.run(debug=True)
```
在以上代码中,我们首先使用csv库读取CSV文件中的数据,并将每条微博的点赞数和时间信息存储到一个列表中。然后使用datetime库将时间信息转换成月份,并将每个月的点赞数数据分别存储到一个字典中。接着使用matplotlib库将字典中的数据转换成趋势图,并将所有趋势图保存到本地。最后使用Flask框架创建一个网页,并将趋势图嵌入到网页中。
阅读全文
相关推荐
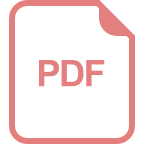
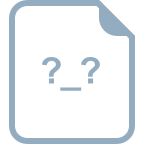

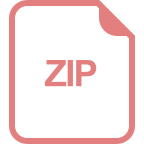
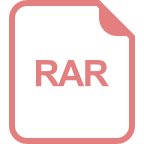
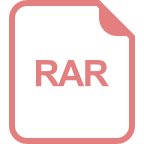
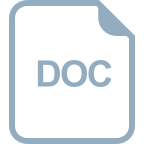
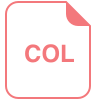
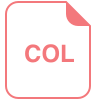
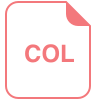
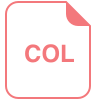
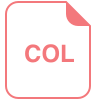
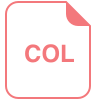
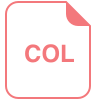
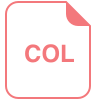
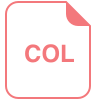
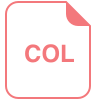
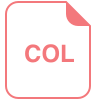
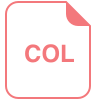