public void in(String key, Object... value) { if(null==inMap){ inMap=new HashMap<String, List<Object>>(5); } List<Object> list=new ArrayList<Object>(); if(value!=null&&value.length>0){ for(int i=0;i<value.length;i++){ if(value[i] instanceof List) { list.addAll((List<Object>)value[i]); } else { list.add(value[i]); } } } inMap.put(key, list); }
时间: 2024-02-14 07:35:38 浏览: 21
这是一个Java代码段,该代码实现了一个in查询操作的方法。方法的输入参数包括一个字符串类型的key和一个可变长度的Object类型的value数组。
该方法中,首先判断inMap是否为空,如果是则创建一个HashMap类型的inMap,用于存储查询条件。然后,将传入的value数组中的元素逐一添加到一个ArrayList类型的list中,如果value数组中的元素是List类型的,则将List中的元素全部添加到list中。最后,将key和list作为键值对存储到inMap中。
该方法的作用是将多个值作为in查询的条件进行查询。
相关问题
在Java中用Dom4j把这一段xml内容转成Map对象:<?xml version="1.0" encoding="UTF-8"?> <java version="1.6.0_26" class="java.beans.XMLDecoder"> <object class="java.util.HashMap"> <void method="put"> <string>att_20160719180419281NEk9</string> <string>cx</string> </void> <void method="put"> <string>att_20160511094248589ZKYq</string> <string>cnmm</string> </void> <void method="put"> <string>att_20161018153614188aTus</string> <string>zsx;fj</string> </void> <void method="put"> <string>att_20160422110906494XuEf</string> <null/> </void> <void method="put"> <string>att_20160511130210612UULE</string> <string>fjyxapp;jjgjapp;wx;jd;dxjg;tb;wsjy</string> </void> <void method="put"> <string>att_20160719180542840LnFm</string> <string></string> </void> <void method="put"> <string>att_20160511130025203ssle</string> <string>zdgsszta</string> </void> <void method="put"> <string>att_20160810131359346N0ra</string> <string>szjys</string> </void> <void method="put"> <string>att_20160511130031927equg</string> <string></string> </void> <void method="put"> <string>att_20160422110456036qp7Y</string> <null/> </void> <void method="put"> <string>att_20160422110543585f7iI</string> <string>cpjbxx</string> </void> </object> </java>
您可以使用以下代码将该XML转换为Map对象:
```java
import java.io.StringReader;
import java.util.HashMap;
import java.util.Map;
import org.dom4j.Document;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
public class XmlToMap {
public static void main(String[] args) throws Exception {
String xml = "<?xml version=\"1.0\" encoding=\"UTF-8\"?> <java version=\"1.6.0_26\" class=\"java.beans.XMLDecoder\"> <object class=\"java.util.HashMap\"> <void method=\"put\"> <string>att_20160719180419281NEk9</string> <string>cx</string> </void> <void method=\"put\"> <string>att_20160511094248589ZKYq</string> <string>cnmm</string> </void> <void method=\"put\"> <string>att_20161018153614188aTus</string> <string>zsx;fj</string> </void> <void method=\"put\"> <string>att_20160422110906494XuEf</string> <null/> </void> <void method=\"put\"> <string>att_20160511130210612UULE</string> <string>fjyxapp;jjgjapp;wx;jd;dxjg;tb;wsjy</string> </void> <void method=\"put\"> <string>att_20160719180542840LnFm</string> <string></string> </void> <void method=\"put\"> <string>att_20160511130025203ssle</string> <string>zdgsszta</string> </void> <void method=\"put\"> <string>att_20160810131359346N0ra</string> <string>szjys</string> </void> <void method=\"put\"> <string>att_20160511130031927equg</string> <string></string> </void> <void method=\"put\"> <string>att_20160422110456036qp7Y</string> <null/> </void> <void method=\"put\"> <string>att_20160422110543585f7iI</string> <string>cpjbxx</string> </void> </object> </java>";
Map<String, String> map = xmlToMap(xml);
System.out.println(map);
}
public static Map<String, String> xmlToMap(String xml) throws Exception {
Map<String, String> map = new HashMap<>();
SAXReader reader = new SAXReader();
Document document = reader.read(new StringReader(xml));
Element root = document.getRootElement().element("object");
for (Element element : root.elements("void")) {
Element keyElement = element.element("string");
Element valueElement = element.elements().get(1);
String key = keyElement.getText();
String value = valueElement == null ? null : valueElement.getText();
map.put(key, value);
}
return map;
}
}
```
该代码使用Dom4j库解析XML,并将其转换为Map对象。输出结果为:
```
{att_20160719180419281NEk9=cx, att_20160511094248589ZKYq=cnmm, att_20161018153614188aTus=zsx;fj, att_20160422110906494XuEf=null, att_20160511130210612UULE=fjyxapp;jjgjapp;wx;jd;dxjg;tb;wsjy, att_20160719180542840LnFm=, att_20160511130025203ssle=zdgsszta, att_20160810131359346N0ra=szjys, att_20160511130031927equg=, att_20160422110456036qp7Y=null, att_20160422110543585f7iI=cpjbxx}
```
public static Map<String, Object> clzToMap(Class clz) { Map<String, Object> user = new HashMap<>(); for (Field declaredField : clz.getDeclaredFields()) { user.put(declaredField.getName(),null); } return user; }这个方法有什么可以改进的吗
这个方法已经可以实现将类的所有属性名作为Map的key,value为null。但是有以下几点可以改进:
1. 该方法没有考虑到类的继承关系,如果该类继承了其他类,那么只能获取该类的属性而无法获取父类的属性。可以使用`getFields()`方法获取该类及其父类的所有公共属性,使用`getDeclaredFields()`方法获取该类的所有属性(包括私有属性)。
2. 该方法没有考虑到类的属性类型,如果属性是引用类型,那么将其值置为null可能会导致空指针异常。可以根据属性类型设置默认值,例如对于字符串类型可以将其值置为空字符串。
3. 该方法返回的Map的value都是null,可能会导致一些问题。可以根据业务需求设置属性的默认值,例如对于数字类型可以将其值置为0。
综上所述,以下是一个改进后的方法:
```java
import java.lang.reflect.Field;
import java.util.HashMap;
import java.util.Map;
public class ClassToMapExample {
public static void main(String[] args) {
Map<String, Object> map = clzToMap(MyClass.class);
System.out.println(map); // 输出:{booleanField=false, intField=0, stringField=}
}
public static Map<String, Object> clzToMap(Class<?> clz) {
Map<String, Object> map = new HashMap<>();
Field[] fields = clz.getFields();
for (Field field : fields) {
map.put(field.getName(), getDefaultValue(field.getType()));
}
fields = clz.getDeclaredFields();
for (Field field : fields) {
field.setAccessible(true);
map.put(field.getName(), getDefaultValue(field.getType()));
}
return map;
}
private static Object getDefaultValue(Class<?> type) {
if (type == String.class) {
return "";
} else if (type == Integer.class || type == int.class ||
type == Short.class || type == short.class ||
type == Byte.class || type == byte.class) {
return 0;
} else if (type == Long.class || type == long.class) {
return 0L;
} else if (type == Float.class || type == float.class) {
return 0.0f;
} else if (type == Double.class || type == double.class) {
return 0.0;
} else if (type == Boolean.class || type == boolean.class) {
return false;
} else {
return null;
}
}
private static class MyClass {
public String stringField;
private int intField;
protected boolean booleanField;
}
}
```
改进后的方法可以处理类的继承关系、属性类型,并根据类型设置默认值。
相关推荐
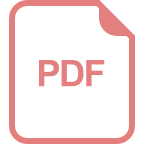
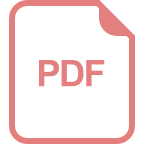
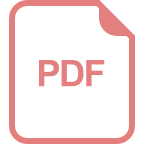












