c++中#include <algorithm>是什么意思
时间: 2024-05-31 08:10:20 浏览: 24
`#include <algorithm>` 是 C++ 标准库中常用的头文件之一,它包含了许多算法函数和结构体,可以用于对容器(如数组、向量、字符串等)进行排序、查找、复制、合并等操作。
例如,`sort()` 函数就是在 `<algorithm>` 头文件中定义的,可以用于对数组或向量进行排序。还有 `find()`、`binary_search()`、`copy()`、`merge()` 等函数也都是在该头文件中定义的。
因此,当我们需要使用其中的算法函数时,需要包含 `<algorithm>` 头文件,以便调用其中的函数。
相关问题
#include<algorithm>为何报错
在C++中,`#include <algorithm>`通常不会直接报错,除非你的编译器在查找头文件时遇到了问题,或者你没有正确地设置包含路径(如头文件不是标准库的一部分,或者不在编译器预设的搜索路径中)。
如果编译器报错,可能的原因有:
1. **找不到头文件**: 你需要确保`<algorithm>`是C++标准库的一部分,它通常位于`std`命名空间中。如果你使用的是较旧的编译器或者自定义的第三方库,可能需要手动添加包含路径或更新库版本。
2. **命名空间问题**: 如果你在代码中没有使用`std::`前缀来引用`<algorithm>`中的函数,可能会因为命名空间冲突而导致错误。C++11及以后版本推荐使用`std::`前缀。
3. **编译选项**: 有些情况下,你可能需要添加`-std=c++xx`或`-std=gnu++xx`等编译选项来指定C++标准版本,特别是当你的项目依赖于新特性时。
4. **代码位置**: 请确认`#include <algorithm>`是在其他必要的`#include`之后,因为有些库可能依赖于`<algorithm>`。
如果能提供具体的错误信息,我能更好地帮助你定位问题。如果你尝试编译后遇到类似这样的错误:
```
error: no matching function for call to 'std::function<void()>::function()'
```
这可能意味着你的编译器不支持`<algorithm>`中的某个功能,或者是`<function>`而不是`<algorithm>`导致的问题。
#include<algorithm>
`#include<algorithm>` 是 C++ 标准库中的一个预处理器指令,它包含了大量用于算法的头文件。C++ 标准库中的 `<algorithm>` 非常重要,它提供了许多实用的函数和迭代器操作,用于处理集合(如向量、列表或数组)中的元素。这些函数涵盖了排序(sort)、查找(find)、查找范围(find_if)、遍历(copy)、交换元素(swap)等等。
- 一些常用的 `<algorithm>` 函数例子包括:
- `std::sort`: 对容器中的元素进行排序。
- `std::find`: 查找容器中是否存在特定元素的第一个匹配项。
- `std::count`: 计算容器中某个元素出现的次数。
- `std::transform`: 应用一个函数到容器的所有元素上。
使用 `<algorithm>` 时,通常配合迭代器一起工作,因为迭代器提供了一种安全和高效的方法来访问和修改容器的内容。
相关推荐
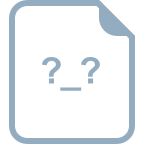
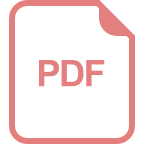
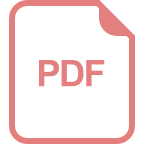












