mvc 中使用对表格进行查询的方法有
时间: 2024-02-24 19:58:08 浏览: 161
在 ASP.NET MVC 中,我们可以通过使用 LINQ 查询语句和表格插件来实现对表格进行查询的功能。以下是一个示例代码:
Model:
```csharp
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
public decimal Price { get; set; }
}
```
Controller:
```csharp
public class ProductController : Controller
{
private readonly ApplicationDbContext db = new ApplicationDbContext();
public ActionResult Index()
{
return View();
}
public ActionResult Search(string searchString)
{
var products = db.Products.Where(p => p.Name.Contains(searchString)).ToList();
return Json(products, JsonRequestBehavior.AllowGet);
}
}
```
Index View:
```html
@{
ViewBag.Title = "Product List";
}
<h2>@ViewBag.Title</h2>
<div>
<input type="text" id="searchString" placeholder="Search...">
<button id="searchButton">Search</button>
</div>
<table id="productTable">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Description</th>
<th>Price</th>
</tr>
</thead>
<tbody>
@foreach (var product in Model)
{
<tr>
<td>@product.Id</td>
<td>@product.Name</td>
<td>@product.Description</td>
<td>@product.Price</td>
</tr>
}
</tbody>
</table>
@section Scripts {
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdn.datatables.net/1.11.3/js/jquery.dataTables.min.js"></script>
<script>
$(document).ready(function () {
var table = $('#productTable').DataTable({
searching: false,
paging: false,
info: false,
columns: [
{ data: 'Id' },
{ data: 'Name' },
{ data: 'Description' },
{ data: 'Price' }
]
});
$('#searchButton').click(function () {
var searchString = $('#searchString').val();
$.ajax({
url: '/Product/Search',
type: 'GET',
data: { searchString: searchString },
success: function (data) {
table.clear().rows.add(data).draw();
}
});
});
});
</script>
}
```
上述代码中,我们在控制器中编写了一个 Search 方法,该方法接收一个搜索关键字并返回符合条件的 Product 列表数据。在 Index 视图中,我们使用了 DataTables 插件来渲染表格,并在 JavaScript 中编写了一个 AJAX 请求,将搜索关键字传递给服务器进行查询,并将查询结果重新渲染到表格中。这里通过 table.clear().rows.add(data).draw() 方法来实现表格的重新渲染。
使用 DataTables 插件可以使表格具有更好的交互性和可定制性,同时,我们通过使用 AJAX 请求来实现无需刷新页面即可进行表格数据查询的功能,提高了用户体验。
阅读全文
相关推荐





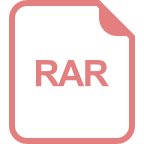









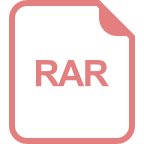

