jschutil 判断文件是否存在
时间: 2023-10-27 22:07:22 浏览: 109
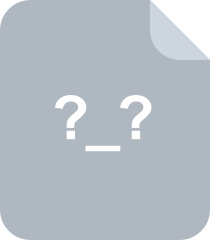
js判定文件是否存在

你可以使用 JSchUtil 库中的 `SftpClient` 类来判断远程服务器上的文件是否存在。以下是一个示例代码:
```java
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSchException;
import com.jcraft.jsch.Session;
import java.io.IOException;
public class SftpUtil {
private Session session;
private ChannelSftp channelSftp;
public SftpUtil(Session session) throws JSchException {
this.session = session;
this.channelSftp = (ChannelSftp) session.openChannel("sftp");
this.channelSftp.connect();
}
public boolean exists(String remoteFilePath) throws SftpException {
try {
channelSftp.stat(remoteFilePath);
return true;
} catch (SftpException e) {
if (e.id == ChannelSftp.SSH_FX_NO_SUCH_FILE) {
return false;
} else {
throw e;
}
}
}
public void disconnect() {
if (channelSftp != null) {
channelSftp.disconnect();
}
if (session != null) {
session.disconnect();
}
}
}
```
使用示例:
```java
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.JSchException;
import com.jcraft.jsch.Session;
public class Main {
public static void main(String[] args) throws JSchException, SftpException {
JSch jSch = new JSch();
Session session = jSch.getSession("username", "hostname", 22);
session.setPassword("password");
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
SftpUtil sftpUtil = new SftpUtil(session);
boolean exists = sftpUtil.exists("/path/to/remote/file");
System.out.println(exists);
sftpUtil.disconnect();
}
}
```
这个示例代码中,我们通过调用 `exists` 方法来判断远程服务器上的文件是否存在。如果文件存在,则返回 `true`,否则返回 `false`。
阅读全文
相关推荐
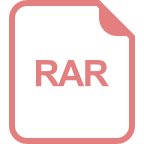
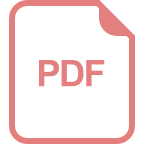
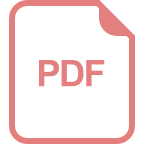
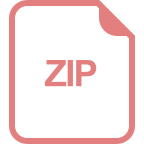
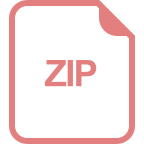
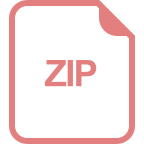
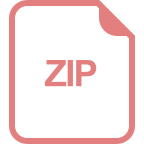
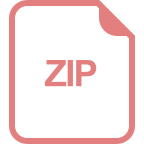
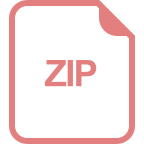
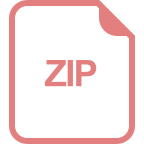
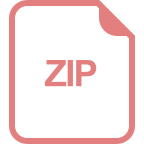
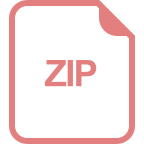
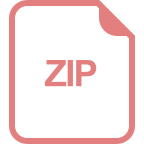