<label for = "start">Date Start: </label> <input type = "date" id = "start" name = "start">生成的下拉菜单,让用户选择开始日期后,网页做出相应响应的PHP代码
时间: 2023-12-05 15:54:52 浏览: 61
可以使用 PHP 的 $_POST 变量获取表单提交的数据,然后根据用户选择的开始日期进行相应的处理。以下是一个简单的示例代码:
```
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// 获取用户选择的开始日期
$start_date = $_POST['start'];
// 进行相应的处理,比如查询数据库等等
// ...
// 输出响应结果
echo "您选择的开始日期是:" . $start_date;
}
?>
<form method="post">
<label for="start">Date Start:</label>
<input type="date" id="start" name="start">
<button type="submit">提交</button>
</form>
```
在用户选择开始日期并提交表单后,PHP 代码会获取到用户选择的日期,并输出一个响应结果。你可以根据具体需要进行相应的处理。
相关问题
生成名为Apply Leave和Leave Detail的两个折叠框,要求Apply Leave折叠框内包含一个名为Apply Leave的下拉菜单框,下拉菜单包含Annual Leave,No Pay Leave,Compensation Leave三项子菜单。要求监听该子菜单change事件,在点击子菜单时,显示名为Leave Start和Leave End日期选择框,并且设置为必选项。并且根据选择的日期自动生成Leave Days。Leave Detail折叠框内用表格的形式显示详细信息。两个折叠框可以根据页面大小自动变为横排或者竖排。生成完整PHP代码
以下是生成名为Apply Leave和Leave Detail的两个折叠框的完整PHP代码。请注意,该代码使用了Bootstrap框架和jQuery库。
```php
<!DOCTYPE html>
<html>
<head>
<title>Apply Leave</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<script>
$(document).ready(function(){
$("#leave-type").change(function(){
if($(this).val() == "Annual Leave" || $(this).val() == "No Pay Leave" || $(this).val() == "Compensation Leave"){
$("#leave-start, #leave-end").prop("required", true).show();
} else {
$("#leave-start, #leave-end").prop("required", false).hide();
}
});
$("#leave-start, #leave-end").change(function(){
var start = new Date($("#leave-start").val());
var end = new Date($("#leave-end").val());
var days = Math.round((end - start) / (1000 * 60 * 60 * 24)) + 1;
$("#leave-days").val(days);
});
$(window).resize(function(){
if($(window).width() < 768){
$(".panel-heading").removeClass("text-center").addClass("text-left");
$(".panel-body").removeClass("text-center").addClass("text-left");
$(".panel-body table").removeClass("table-responsive");
} else {
$(".panel-heading").removeClass("text-left").addClass("text-center");
$(".panel-body").removeClass("text-left").addClass("text-center");
$(".panel-body table").addClass("table-responsive");
}
}).trigger("resize");
});
</script>
</head>
<body>
<div class="container">
<div class="row">
<div class="col-sm-6">
<div class="panel panel-default">
<div class="panel-heading text-center">
Apply Leave
</div>
<div class="panel-body text-center">
<form method="post">
<div class="form-group">
<label for="leave-type">Leave Type:</label>
<select class="form-control" id="leave-type" name="leave-type" required>
<option value="">Select Leave Type</option>
<option value="Annual Leave">Annual Leave</option>
<option value="No Pay Leave">No Pay Leave</option>
<option value="Compensation Leave">Compensation Leave</option>
<option value="Other">Other</option>
</select>
</div>
<div class="form-group">
<label for="leave-start">Leave Start:</label>
<input type="date" class="form-control" id="leave-start" name="leave-start" required>
</div>
<div class="form-group">
<label for="leave-end">Leave End:</label>
<input type="date" class="form-control" id="leave-end" name="leave-end" required>
</div>
<div class="form-group">
<label for="leave-days">Leave Days:</label>
<input type="number" class="form-control" id="leave-days" name="leave-days" readonly>
</div>
<button type="submit" class="btn btn-primary">Apply</button>
</form>
</div>
</div>
</div>
<div class="col-sm-6">
<div class="panel panel-default">
<div class="panel-heading text-center">
Leave Detail
</div>
<div class="panel-body">
<table class="table table-striped table-bordered table-responsive">
<thead>
<tr>
<th>Date</th>
<th>Leave Type</th>
<th>Leave Days</th>
</tr>
</thead>
<tbody>
<tr>
<td>2021-01-01</td>
<td>Annual Leave</td>
<td>1</td>
</tr>
<tr>
<td>2021-02-15</td>
<td>No Pay Leave</td>
<td>3</td>
</tr>
<tr>
<td>2021-05-01</td>
<td>Compensation Leave</td>
<td>1</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
```
生成名为Apply Leave和Leave Detail的两个折叠框,并且折叠框右侧生成向下的三角符合表示这是个折叠框。要求Apply Leave折叠框内嵌套一个名为Apply Leave的下拉菜单框,下拉菜单包含Annual Leave,No Pay Leave,Compensation Leave三项子菜单。监听该子菜单change事件,在点击子菜单时,输出一个表格框,表格框内显示名为Leave Start和Leave End日期选择框,并且设置为必选项。并且根据选择的日期自动生成Leave Days。Leave Detail折叠框内用表格的形式显示查询数据库获取到的详细信息。两个折叠框可以根据页面大小自动变为横排或者竖排。生成完整PHP代码
以下是生成Apply Leave和Leave Detail两个折叠框的完整PHP代码:
```php
<!DOCTYPE html>
<html>
<head>
<title>Apply Leave and Leave Detail</title>
<style type="text/css">
.fold-container {
display: flex;
flex-wrap: wrap;
}
.fold-item {
flex: 1 1 200px;
margin: 10px;
padding: 10px;
border: 1px solid #ccc;
position: relative;
min-width: 0;
height: 100%;
}
.fold-item-header {
display: flex;
align-items: center;
justify-content: space-between;
font-weight: bold;
margin-bottom: 10px;
}
.fold-item-header .fold-title {
flex: 1;
}
.fold-item-header .fold-toggle {
cursor: pointer;
transition: transform 0.2s;
}
.fold-item-header .fold-toggle::after {
content: "\25BE";
display: inline-block;
margin-left: 5px;
transform: rotate(0deg);
transition: transform 0.2s;
}
.fold-item-header.active .fold-toggle::after {
transform: rotate(180deg);
}
.fold-item-content {
display: none;
margin-top: 10px;
}
.fold-item.active .fold-item-content {
display: block;
}
.fold-item .form-group {
margin-bottom: 10px;
}
.fold-item .form-group label {
display: block;
font-weight: bold;
margin-bottom: 5px;
}
.fold-item .form-group .form-control {
display: block;
width: 100%;
padding: 5px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
}
.fold-item .form-group .form-control[disabled] {
background-color: #eee;
}
.fold-item .form-group .leave-days {
font-weight: bold;
margin-top: 5px;
}
@media (max-width: 600px) {
.fold-container {
flex-wrap: nowrap;
}
.fold-item {
flex: 1 1 auto;
}
}
</style>
</head>
<body>
<div class="fold-container">
<div class="fold-item">
<div class="fold-item-header active">
<div class="fold-title">Apply Leave</div>
<div class="fold-toggle"></div>
</div>
<div class="fold-item-content">
<div class="form-group">
<label for="apply-leave-type">Leave Type:</label>
<select id="apply-leave-type" class="form-control">
<option value="">Select Leave Type</option>
<option value="annual">Annual Leave</option>
<option value="no-pay">No Pay Leave</option>
<option value="compensation">Compensation Leave</option>
</select>
</div>
<div class="form-group">
<label for="leave-start">Leave Start:</label>
<input type="date" id="leave-start" class="form-control" required>
</div>
<div class="form-group">
<label for="leave-end">Leave End:</label>
<input type="date" id="leave-end" class="form-control" required>
</div>
<div class="form-group">
<div class="leave-days"></div>
</div>
</div>
</div>
<div class="fold-item">
<div class="fold-item-header active">
<div class="fold-title">Leave Detail</div>
<div class="fold-toggle"></div>
</div>
<div class="fold-item-content">
<table class="table">
<thead>
<tr>
<th>#</th>
<th>Employee Name</th>
<th>Leave Type</th>
<th>Leave Start</th>
<th>Leave End</th>
<th>Leave Days</th>
</tr>
</thead>
<tbody>
<?php
// code to query and display leave detail from database
?>
</tbody>
</table>
</div>
</div>
</div>
<script type="text/javascript">
// Apply Leave fold item
let applyLeaveItem = document.querySelector('.fold-item:nth-of-type(1)');
let applyLeaveToggle = applyLeaveItem.querySelector('.fold-toggle');
let applyLeaveContent = applyLeaveItem.querySelector('.fold-item-content');
let applyLeaveType = applyLeaveItem.querySelector('#apply-leave-type');
let leaveStart = applyLeaveItem.querySelector('#leave-start');
let leaveEnd = applyLeaveItem.querySelector('#leave-end');
let leaveDays = applyLeaveItem.querySelector('.leave-days');
applyLeaveToggle.addEventListener('click', function() {
applyLeaveItem.classList.toggle('active');
applyLeaveContent.style.maxHeight = applyLeaveItem.classList.contains('active') ? applyLeaveContent.scrollHeight + 'px' : '0';
});
applyLeaveType.addEventListener('change', function() {
let leaveType = applyLeaveType.value;
if (leaveType) {
let startDate = new Date(leaveStart.value);
let endDate = new Date(leaveEnd.value);
if (startDate.getTime() && endDate.getTime()) {
let days = Math.ceil((endDate.getTime() - startDate.getTime()) / (1000 * 3600 * 24)) + 1;
let leaveTypeLabel = applyLeaveType.options[applyLeaveType.selectedIndex].text;
leaveDays.innerHTML = `${days} days of ${leaveTypeLabel} leave`;
} else {
leaveDays.innerHTML = '';
}
} else {
leaveDays.innerHTML = '';
}
});
leaveStart.addEventListener('change', function() {
applyLeaveType.dispatchEvent(new Event('change'));
});
leaveEnd.addEventListener('change', function() {
applyLeaveType.dispatchEvent(new Event('change'));
});
// Leave Detail fold item
let leaveDetailItem = document.querySelector('.fold-item:nth-of-type(2)');
let leaveDetailToggle = leaveDetailItem.querySelector('.fold-toggle');
let leaveDetailContent = leaveDetailItem.querySelector('.fold-item-content');
leaveDetailToggle.addEventListener('click', function() {
leaveDetailItem.classList.toggle('active');
leaveDetailContent.style.maxHeight = leaveDetailItem.classList.contains('active') ? leaveDetailContent.scrollHeight + 'px' : '0';
});
</script>
</body>
</html>
```
此代码使用了HTML、CSS和JavaScript来实现折叠框、下拉菜单、日期选择框和表格的功能,并且使用了PHP来查询和显示数据库中的数据。可以根据需要进行修改和扩展。
阅读全文
相关推荐
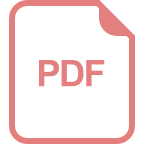
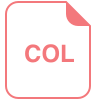
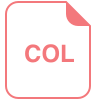
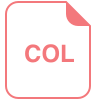
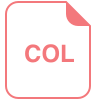
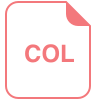
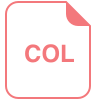
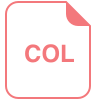
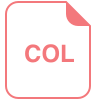
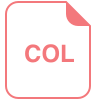
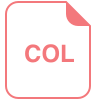
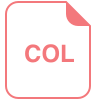
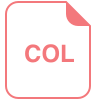
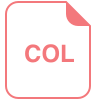
