vue 项目中 echarts树图 如何实现点击节点调用接口动态获取下一节点数据 全部案例代码
时间: 2023-11-28 12:48:44 浏览: 39
以下是一个简单的实现:
1. 在 Vue 中引入 echarts
```
import echarts from 'echarts/lib/echarts';
import 'echarts/lib/chart/tree';
import 'echarts/lib/component/tooltip';
import 'echarts/lib/component/title';
```
2. 在组件中定义一个 echarts 实例和数据
```
<template>
<div ref="chart" style="height: 500px;"></div>
</template>
<script>
export default {
data() {
return {
chart: null, // echarts 实例
treeData: [], // 树图数据
};
},
mounted() {
this.initChart();
this.getTreeData();
},
methods: {
initChart() {
this.chart = echarts.init(this.$refs.chart);
// 配置 echarts 树图的 option
const option = {
series: [
{
type: 'tree',
data: [],
// 点击节点时触发
emphasis: {
focus: 'descendant',
},
// 其他配置...
},
],
};
this.chart.setOption(option);
},
getTreeData() {
// 调用接口获取树图数据
api.getTreeData().then((res) => {
this.treeData = res.data;
// 更新 echarts 树图数据
this.chart.setOption({
series: [
{
data: [this.treeData],
},
],
});
});
},
},
};
</script>
```
3. 在点击节点时调用接口获取下一节点数据,并更新 echarts 树图数据
```
<template>
<div ref="chart" style="height: 500px;"></div>
</template>
<script>
export default {
data() {
return {
chart: null, // echarts 实例
treeData: [], // 树图数据
};
},
mounted() {
this.initChart();
this.getTreeData();
},
methods: {
initChart() {
this.chart = echarts.init(this.$refs.chart);
// 配置 echarts 树图的 option
const option = {
series: [
{
type: 'tree',
data: [],
// 点击节点时触发
emphasis: {
focus: 'descendant',
},
// 其他配置...
},
],
};
this.chart.setOption(option);
// 监听 echarts 树图的点击事件
this.chart.on('click', this.handleNodeClick);
},
getTreeData(id) {
// 调用接口获取树图数据
api.getTreeData(id).then((res) => {
this.treeData = res.data;
// 更新 echarts 树图数据
this.chart.setOption({
series: [
{
data: [this.treeData],
},
],
});
});
},
handleNodeClick(params) {
const { id } = params.data;
this.getTreeData(id);
},
},
};
</script>
```
4. 完整代码示例
```
<template>
<div ref="chart" style="height: 500px;"></div>
</template>
<script>
import echarts from 'echarts/lib/echarts';
import 'echarts/lib/chart/tree';
import 'echarts/lib/component/tooltip';
import 'echarts/lib/component/title';
export default {
data() {
return {
chart: null, // echarts 实例
treeData: [], // 树图数据
};
},
mounted() {
this.initChart();
this.getTreeData();
},
methods: {
initChart() {
this.chart = echarts.init(this.$refs.chart);
// 配置 echarts 树图的 option
const option = {
series: [
{
type: 'tree',
data: [],
// 点击节点时触发
emphasis: {
focus: 'descendant',
},
// 其他配置...
},
],
};
this.chart.setOption(option);
// 监听 echarts 树图的点击事件
this.chart.on('click', this.handleNodeClick);
},
getTreeData(id) {
// 调用接口获取树图数据
api.getTreeData(id).then((res) => {
this.treeData = res.data;
// 更新 echarts 树图数据
this.chart.setOption({
series: [
{
data: [this.treeData],
},
],
});
});
},
handleNodeClick(params) {
const { id } = params.data;
this.getTreeData(id);
},
},
};
</script>
```
相关推荐
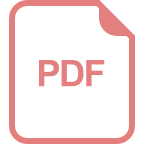
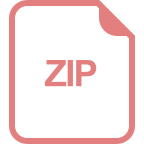
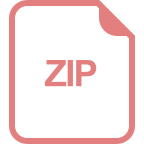














