用java简单文本加解密程序
时间: 2024-03-03 22:49:40 浏览: 16
以下是一个简单的文本加解密程序的Java代码,使用的是Caesar Cipher算法:
```java
import java.util.Scanner;
public class TextEncryption {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the text to encrypt: ");
String text = scanner.nextLine();
System.out.print("Enter the encryption key (an integer between 1 and 26): ");
int key = scanner.nextInt();
String encryptedText = encrypt(text, key);
System.out.println("Encrypted text: " + encryptedText);
String decryptedText = decrypt(encryptedText, key);
System.out.println("Decrypted text: " + decryptedText);
}
public static String encrypt(String text, int key) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < text.length(); i++) {
char ch = text.charAt(i);
if (ch >= 'a' && ch <= 'z') {
ch = (char) ((ch + key - 'a') % 26 + 'a');
} else if (ch >= 'A' && ch <= 'Z') {
ch = (char) ((ch + key - 'A') % 26 + 'A');
}
result.append(ch);
}
return result.toString();
}
public static String decrypt(String text, int key) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < text.length(); i++) {
char ch = text.charAt(i);
if (ch >= 'a' && ch <= 'z') {
ch = (char) ((ch - key - 'a' + 26) % 26 + 'a');
} else if (ch >= 'A' && ch <= 'Z') {
ch = (char) ((ch - key - 'A' + 26) % 26 + 'A');
}
result.append(ch);
}
return result.toString();
}
}
```
该程序使用了Scanner类从控制台读取用户输入。用户需要输入要加密的文本和加密密钥。加密函数和解密函数分别对文本进行加密和解密,使用了Caesar Cipher算法,该算法是一种简单的替换加密算法,将每个字符替换成其后面的第k个字符。在该程序中,密钥k是一个整数,表示替换的位数。程序通过对每个字符进行偏移来加密和解密文本,如果字符是小写字母,则将其替换成a到z中后面第k个字母,如果字符是大写字母,则将其替换成A到Z中后面第k个字母。
相关推荐
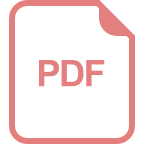
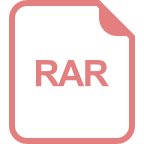
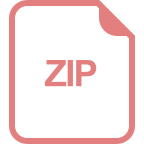
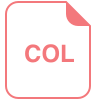
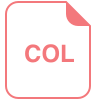
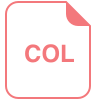
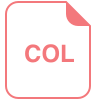
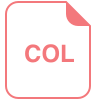










