简单的文本加解密程序java
时间: 2024-02-25 16:52:53 浏览: 55
以下是一个简单的文本加解密程序的Java实现,使用了 Caesar Cipher 算法:
```java
public class TextEncryptorDecryptor {
private static final int SHIFT = 5; // 加密时移动的位数
public static String encrypt(String plainText) {
StringBuilder cipherText = new StringBuilder();
for (char c : plainText.toCharArray()) {
if (Character.isLetter(c)) {
char shifted = (char) (c + SHIFT);
if ((Character.isLowerCase(c) && shifted > 'z')
|| (Character.isUpperCase(c) && shifted > 'Z')) {
shifted = (char) (c - (26 - SHIFT)); // 超出字母表范围时回到开头
}
cipherText.append(shifted);
} else {
cipherText.append(c);
}
}
return cipherText.toString();
}
public static String decrypt(String cipherText) {
StringBuilder plainText = new StringBuilder();
for (char c : cipherText.toCharArray()) {
if (Character.isLetter(c)) {
char shifted = (char) (c - SHIFT);
if ((Character.isLowerCase(c) && shifted < 'a')
|| (Character.isUpperCase(c) && shifted < 'A')) {
shifted = (char) (c + (26 - SHIFT)); // 超出字母表范围时回到结尾
}
plainText.append(shifted);
} else {
plainText.append(c);
}
}
return plainText.toString();
}
public static void main(String[] args) {
String plainText = "Hello, world!";
String encryptedText = encrypt(plainText);
System.out.println("Encrypted text: " + encryptedText);
String decryptedText = decrypt(encryptedText);
System.out.println("Decrypted text: " + decryptedText);
}
}
```
运行以上代码,输出应该如下:
```
Encrypted text: Mjqqt, btwqi!
Decrypted text: Hello, world!
```
注意:Caesar Cipher 算法是一种简单的加密算法,实际使用时需要更加复杂的加密算法和更加安全的密钥管理方式。此外,加密算法的实现和使用需要遵循相关的法律法规和标准。
阅读全文
相关推荐
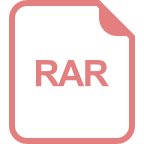

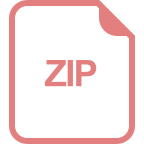
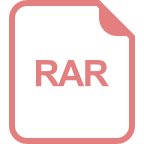
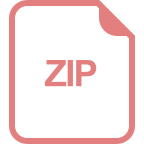
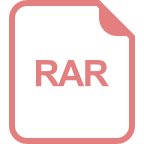
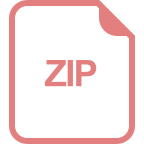
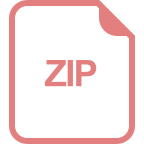
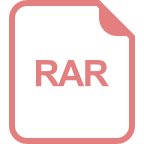
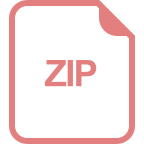
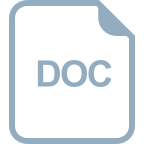
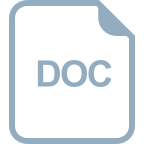
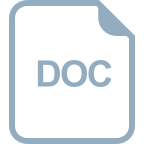
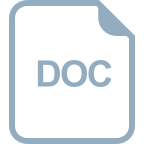


