用Java语言设计并实现DES加密解密程序,利用实现的DES加密解密程序分别对字符串、文本、图像等数据进行加密和解密。
时间: 2024-01-24 20:20:37 浏览: 115
以下是Java语言实现DES加密解密程序的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
public class DesUtil {
private static final String ALGORITHM = "DES";
public static SecretKey generateKey() throws NoSuchAlgorithmException {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
return keyGenerator.generateKey();
}
public static byte[] encrypt(byte[] data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
public static void encryptFile(String inputFile, String outputFile, SecretKey secretKey) throws Exception {
byte[] data = Files.readAllBytes(Paths.get(inputFile));
byte[] encryptedData = encrypt(data, secretKey);
FileOutputStream outputStream = new FileOutputStream(outputFile);
outputStream.write(encryptedData);
outputStream.close();
}
public static void decryptFile(String inputFile, String outputFile, SecretKey secretKey) throws Exception {
byte[] data = Files.readAllBytes(Paths.get(inputFile));
byte[] decryptedData = decrypt(data, secretKey);
FileOutputStream outputStream = new FileOutputStream(outputFile);
outputStream.write(decryptedData);
outputStream.close();
}
public static void main(String[] args) throws Exception {
String data = "Hello, world!";
SecretKey secretKey = generateKey();
byte[] encryptedData = encrypt(data.getBytes(), secretKey);
byte[] decryptedData = decrypt(encryptedData, secretKey);
System.out.println("Original data: " + data);
System.out.println("Encrypted data: " + Base64.getEncoder().encodeToString(encryptedData));
System.out.println("Decrypted data: " + new String(decryptedData));
String inputFile = "test.txt";
String encryptedFile = "test_encrypted.txt";
String decryptedFile = "test_decrypted.txt";
Files.write(Paths.get(inputFile), data.getBytes());
encryptFile(inputFile, encryptedFile, secretKey);
decryptFile(encryptedFile, decryptedFile, secretKey);
String decryptedFileContent = new String(Files.readAllBytes(Paths.get(decryptedFile)));
System.out.println("Original file content: " + data);
System.out.println("Decrypted file content: " + decryptedFileContent);
}
}
```
使用示例:
```java
String data = "Hello, world!";
SecretKey secretKey = DesUtil.generateKey();
byte[] encryptedData = DesUtil.encrypt(data.getBytes(), secretKey);
byte[] decryptedData = DesUtil.decrypt(encryptedData, secretKey);
System.out.println("Original data: " + data);
System.out.println("Encrypted data: " + Base64.getEncoder().encodeToString(encryptedData));
System.out.println("Decrypted data: " + new String(decryptedData));
String inputFile = "test.txt";
String encryptedFile = "test_encrypted.txt";
String decryptedFile = "test_decrypted.txt";
Files.write(Paths.get(inputFile), data.getBytes());
DesUtil.encryptFile(inputFile, encryptedFile, secretKey);
DesUtil.decryptFile(encryptedFile, decryptedFile, secretKey);
String decryptedFileContent = new String(Files.readAllBytes(Paths.get(decryptedFile)));
System.out.println("Original file content: " + data);
System.out.println("Decrypted file content: " + decryptedFileContent);
```
以上代码中,`generateKey()` 方法用于生成一个随机的DES密钥;`encrypt()` 方法用于加密数据;`decrypt()` 方法用于解密数据;`encryptFile()` 和 `decryptFile()` 方法分别用于加密和解密文件。该程序支持对字符串、文本文件和图像文件进行加密和解密。
阅读全文
相关推荐
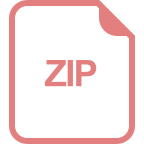
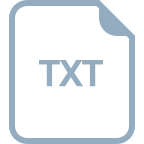
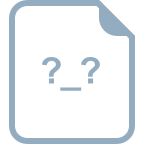
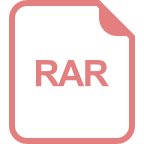
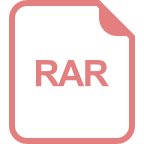
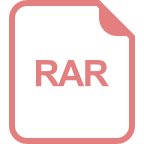
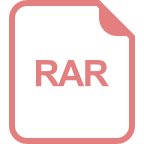
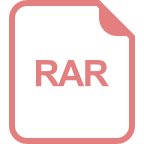
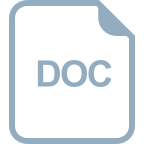
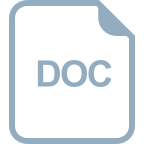
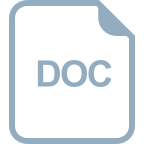
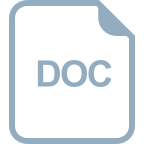