java 实现3DES加密解密
时间: 2023-08-25 15:06:16 浏览: 110
Java中实现3DES加密解密可以使用JCE(Java Cryptography Extension)提供的API。下面是一个简单的示例代码:
```java
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.DESedeKeySpec;
import java.nio.charset.StandardCharsets;
import java.security.spec.KeySpec;
public class TripleDesExample {
public static void main(String[] args) throws Exception {
String plainText = "Hello, world!";
String secretKey = "0123456789ABCDEFGHIGKLMN";
byte[] encryptedBytes = encrypt(plainText, secretKey);
String encryptedText = new String(encryptedBytes, StandardCharsets.UTF_8);
System.out.println("Encrypted text: " + encryptedText);
byte[] decryptedBytes = decrypt(encryptedBytes, secretKey);
String decryptedText = new String(decryptedBytes, StandardCharsets.UTF_8);
System.out.println("Decrypted text: " + decryptedText);
}
public static byte[] encrypt(String plainText, String secretKey) throws Exception {
byte[] plainBytes = plainText.getBytes(StandardCharsets.UTF_8);
byte[] keyBytes = secretKey.getBytes(StandardCharsets.UTF_8);
KeySpec keySpec = new DESedeKeySpec(keyBytes);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DESede");
SecretKey key = keyFactory.generateSecret(keySpec);
Cipher cipher = Cipher.getInstance("DESede");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(plainBytes);
}
public static byte[] decrypt(byte[] encryptedBytes, String secretKey) throws Exception {
byte[] keyBytes = secretKey.getBytes(StandardCharsets.UTF_8);
KeySpec keySpec = new DESedeKeySpec(keyBytes);
SecretKeyFactory keyFactory = SecretKeyFactory.getInstance("DESede");
SecretKey key = keyFactory.generateSecret(keySpec);
Cipher cipher = Cipher.getInstance("DESede");
cipher.init(Cipher.DECRYPT_MODE, key);
return cipher.doFinal(encryptedBytes);
}
}
```
在上面的示例代码中,我们使用了`DESede`算法实现了3DES加密解密。需要注意的是,密钥的长度必须是24个字节(即192位),因此我们在示例中使用了一个24个字符的字符串作为密钥。在实际应用中,我们可以使用更加安全的方式生成密钥,例如使用`KeyGenerator`类生成密钥。
阅读全文
相关推荐
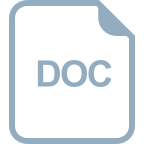
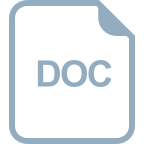
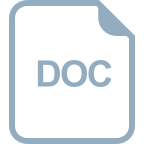
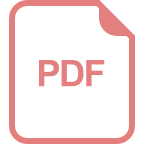
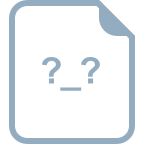
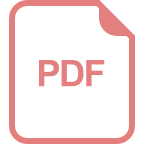
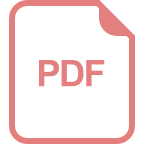
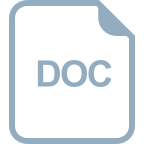
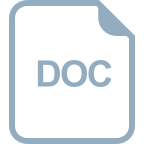
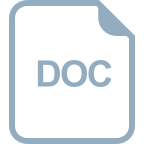
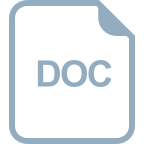

