Java实现DES加密解密代码
时间: 2023-06-04 14:04:54 浏览: 153
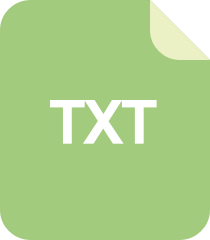
用JAVA实现DES加密解密

好的,我可以为您提供Java实现DES加密解密代码。以下是示例代码:
```
import javax.crypto.*;
import javax.crypto.spec.*;
import java.security.*;
public class DesEncrypter {
Cipher ecipher;
Cipher dcipher;
public DesEncrypter(SecretKey key) throws Exception {
ecipher = Cipher.getInstance("DES");
dcipher = Cipher.getInstance("DES");
ecipher.init(Cipher.ENCRYPT_MODE, key);
dcipher.init(Cipher.DECRYPT_MODE, key);
}
public byte[] encrypt(String str) throws Exception {
// Convert the string to bytes using UTF-8 encoding
byte[] utf8 = str.getBytes("UTF8");
// Encrypt
byte[] enc = ecipher.doFinal(utf8);
return enc;
}
public String decrypt(byte[] enc) throws Exception {
// Decrypt
byte[] utf8 = dcipher.doFinal(enc);
// Convert bytes to string using UTF-8 encoding
String str = new String(utf8, "UTF8");
return str;
}
public static void main(String[] args) throws Exception {
// Generate a key
KeyGenerator keygenerator = KeyGenerator.getInstance("DES");
SecretKey myDesKey = keygenerator.generateKey();
// Initialize the encrypter/decrypter
DesEncrypter encrypter = new DesEncrypter(myDesKey);
// Encrypt
String encrypted = new String(encrypter.encrypt("Hello World"));
// Decrypt
String decrypted = encrypter.decrypt(encrypted.getBytes());
System.out.println("Original String: " + "Hello World");
System.out.println("Encrypted String: " + encrypted);
System.out.println("Decrypted String: " + decrypted);
}
}
```
请注意,此示例代码为简单示例,实际环境中可能需要更严格的安全性措施。
阅读全文
相关推荐

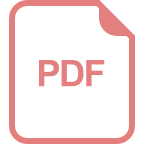
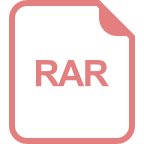
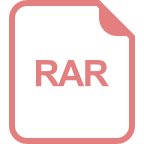
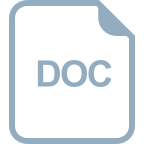
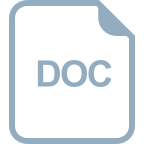
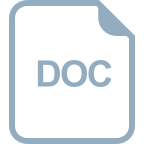
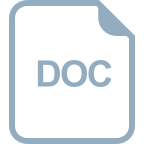
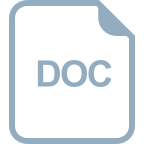
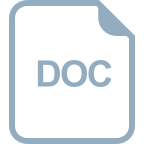


