求一到一百相乘java代码
时间: 2023-07-03 19:30:24 浏览: 81
这里是一到一百相乘的Java代码:
```java
public class MultiplicationTable {
public static void main(String[] args) {
int result = 1;
for (int i = 1; i <= 100; i++) {
result *= i;
}
System.out.println("1到100相乘的结果是:" + result);
}
}
```
这段代码使用了一个for循环来计算1到100的乘积,最后输出结果。
相关问题
java数组相乘代码
Java中数组相乘可以通过循环遍历数组来实现,具体代码如下:
```java
public class ArrayMultiplication {
public static void main(String[] args) {
int[] arr1 = {1, 2, 3};
int[] arr2 = {4, 5, 6};
int[] result = new int[arr1.length];
for (int i = 0; i < arr1.length; i++) {
result[i] = arr1[i] * arr2[i];
}
System.out.println(Arrays.toString(result));
}
}
```
这段代码中,我们定义了两个数组`arr1`和`arr2`,并且定义了一个`result`数组用于存储相乘的结果。然后通过循环遍历`arr1`和`arr2`数组,将对应位置的元素相乘并存储到`result`数组中。最后使用`Arrays.toString()`方法将`result`数组输出到控制台。
MapReduce实现矩阵相乘java代码
以下是使用MapReduce实现矩阵相乘的Java代码:
Mapper类:
```
public class MatrixMapper extends Mapper<LongWritable, Text, Text, Text> {
private Text keyOut = new Text();
private Text valueOut = new Text();
private int k;
@Override
protected void setup(Context context) throws IOException, InterruptedException {
super.setup(context);
Configuration configuration = context.getConfiguration();
k = configuration.getInt("k", 0);
}
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] values = value.toString().split(",");
if (values.length != 3) {
throw new IOException("Invalid input format");
}
int i = Integer.parseInt(values[0]);
int j = Integer.parseInt(values[1]);
int v = Integer.parseInt(values[2]);
if (i < 0 || i >= k || j < 0 || j >= k) {
throw new IOException("Invalid input format");
}
keyOut.set(String.valueOf(j));
valueOut.set("A," + i + "," + v);
context.write(keyOut, valueOut);
keyOut.set(String.valueOf(i));
valueOut.set("B," + j + "," + v);
context.write(keyOut, valueOut);
}
}
```
Reducer类:
```
public class MatrixReducer extends Reducer<Text, Text, Text, IntWritable> {
private IntWritable valueOut = new IntWritable();
private Map<Integer, Integer> aMap = new HashMap<>();
private Map<Integer, Integer> bMap = new HashMap<>();
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
for (Text value : values) {
String[] fields = value.toString().split(",");
if (fields.length != 3) {
throw new IOException("Invalid input format");
}
String matrixName = fields[0];
int i = Integer.parseInt(fields[1]);
int v = Integer.parseInt(fields[2]);
if ("A".equals(matrixName)) {
aMap.put(i, v);
} else if ("B".equals(matrixName)) {
bMap.put(i, v);
} else {
throw new IOException("Invalid input format");
}
}
int sum = 0;
for (Map.Entry<Integer, Integer> entry : aMap.entrySet()) {
int i = entry.getKey();
int a = entry.getValue();
Integer b = bMap.get(i);
if (b != null) {
sum += a * b;
}
}
valueOut.set(sum);
context.write(key, valueOut);
aMap.clear();
bMap.clear();
}
}
```
Driver类:
```
public class MatrixMultiplication {
public static void main(String[] args) throws Exception {
if (args.length != 3) {
System.err.println("Usage: MatrixMultiplication <inputPath> <outputPath> <k>");
System.exit(1);
}
Configuration configuration = new Configuration();
configuration.setInt("k", Integer.parseInt(args[2]));
Job job = Job.getInstance(configuration, "Matrix Multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(MatrixMapper.class);
job.setReducerClass(MatrixReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
这里假设输入文件的格式为每行三个用逗号分隔的数字,分别表示矩阵A或矩阵B的行、列和值。例如,输入文件中的一行可能是`0,0,1`,表示A矩阵的第0行第0列的值为1。
相关推荐
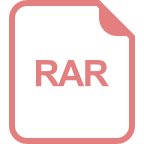
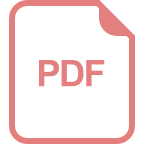








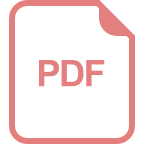
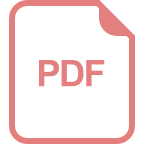
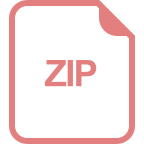
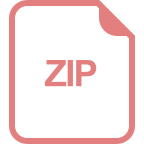