编写一个多线程演示程序,其中一个线程每隔2秒输出6个“hello”,另一个线程每1秒输出5个“good”
时间: 2024-03-11 07:43:39 浏览: 18
以下是一个简单的多线程演示程序,其中一个线程每隔2秒输出6个“hello”,另一个线程每1秒输出5个“good”:
```python
import threading
import time
def say_hello():
for i in range(3):
print("hello " * 6)
time.sleep(2)
def say_good():
for i in range(5):
print("good " * 5)
time.sleep(1)
if __name__ == "__main__":
thread1 = threading.Thread(target=say_hello)
thread2 = threading.Thread(target=say_good)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
```
这个程序使用了 Python 的 threading 模块来创建两个线程。第一个线程每隔2秒输出6个“hello”,使用了 time 模块的 sleep 函数来实现等待。第二个线程每1秒输出5个“good”。
在主程序中,我们创建了两个线程,并使用 start 方法启动它们。然后使用 join 方法等待线程结束,以确保所有线程都执行完毕。最后,程序退出。
相关问题
Java编写一个多线程演示程序,其中一个线程每隔2秒输出6个“hello”,另一个线程每1秒输出5个“good”
以下是一个简单的多线程演示程序,其中一个线程每隔2秒输出6个“hello”,另一个线程每1秒输出5个“good”:
```java
public class MultiThreadDemo {
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
for (int i = 0; i < 3; i++) {
System.out.println("hello hello hello hello hello hello");
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
Thread thread2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
System.out.println("good good good good good");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
thread1.start();
thread2.start();
try {
thread1.join();
thread2.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
这个程序使用了 Java 的线程机制来创建两个线程。第一个线程每隔2秒输出6个“hello”,使用了 Thread.sleep 函数来实现等待。第二个线程每1秒输出5个“good”。
在主程序中,我们创建了两个线程,并使用 start 方法启动它们。然后使用 join 方法等待线程结束,以确保所有线程都执行完毕。最后,程序退出。
编写一个多线程传参的程序
### 回答1:
好的,我来回答一下。编写一个多线程程序需要以下步骤:
1. 导入必要的库和模块,例如`threading`和`time`。
2. 创建一个线程类,继承`threading.Thread`类,并实现`run()`方法。
3. 在主程序中创建线程实例,并传入所需参数。
4. 调用线程的`start()`方法启动线程。
5. 在`run()`方法中编写线程所需的操作,可以使用`time.sleep()`方法模拟一些耗时的操作。
下面是一个简单的多线程程序示例,实现了两个线程同时执行,每个线程循环输出数字,并设置不同的延迟时间:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self, name, delay):
threading.Thread.__init__(self)
self.name = name
self.delay = delay
def run(self):
print("Thread", self.name, "started")
for i in range(5):
print("Thread", self.name, ":", i)
time.sleep(self.delay)
print("Thread", self.name, "finished")
if __name__ == "__main__":
t1 = MyThread("A", 1)
t2 = MyThread("B", 2)
t1.start()
t2.start()
t1.join()
t2.join()
print("All threads finished")
```
在上述示例中,我们首先定义了一个`MyThread`类,继承`threading.Thread`类,实现了`run()`方法,在`run()`方法中实现了循环输出数字,并使用`time.sleep()`方法模拟了1或2秒的延迟。
在主程序中,我们创建了两个线程实例`t1`和`t2`,分别传入线程名称和延迟时间参数,并调用`start()`方法启动线程。
最后使用`join()`方法等待所有线程执行完成,然后输出"All threads finished"。
### 回答2:
多线程是指程序中有多个线程同时运行。在多线程编程中,有时候需要传递参数给线程,让线程可以根据参数的不同执行不同的操作。
下面是一个简单的例子,演示如何编写一个多线程传参的程序:
```python
import threading
def print_message(message):
# 线程要执行的操作
print(message)
def main():
thread1 = threading.Thread(target=print_message, args=("Hello",)) # 创建线程1,并传递参数
thread2 = threading.Thread(target=print_message, args=("World",)) # 创建线程2,并传递参数
thread1.start() # 启动线程1
thread2.start() # 启动线程2
thread1.join() # 等待线程1结束
thread2.join() # 等待线程2结束
if __name__ == "__main__":
main()
```
在上述代码中,我们定义了一个`print_message`函数,用于打印传入的`message`参数。然后,我们创建了两个线程`thread1`和`thread2`,分别传递不同的参数。通过`target`参数指定线程要执行的函数,`args`参数用于传递参数给该函数。
在`main`函数中,我们启动线程1和线程2,并使用`join`方法等待它们的结束。
运行该程序,可以看到输出结果为:
```
Hello
World
```
这表明两个线程分别执行了带有不同参数的`print_message`函数,实现了多线程传参的目的。
### 回答3:
编写一个多线程传参的程序可以使用Python的threading模块来实现。下面是一个示例代码:
```python
import threading
def worker(num):
print("Worker %d started" % num)
# 这里可以进行具体的任务处理
print("Worker %d finished" % num)
if __name__ == "__main__":
threads = []
# 创建并启动多个线程
for i in range(5):
t = threading.Thread(target=worker, args=(i,))
threads.append(t)
t.start()
# 等待所有线程执行完毕
for t in threads:
t.join()
print("All workers finished")
```
在这个例子中,我们定义了一个`worker`函数作为线程的任务函数,它接受一个`num`参数代表线程的编号。我们创建了5个线程,并将线程对象添加到一个列表中。然后,我们逐个启动线程,并等待所有线程执行完毕。
注意要使用`args`参数将参数传递给线程的任务函数,参数必须以元组的形式提供。
希望这个示例代码能帮助你编写多线程传参的程序!
相关推荐
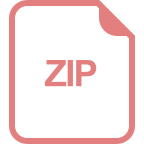
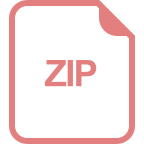
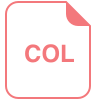
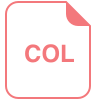
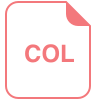
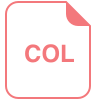
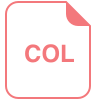







