运用java,利用多线程机制编写程序,输出一个问候语,要求每隔1秒钟输出一个字符。用2种方法分别创建线程。
时间: 2024-03-06 15:49:26 浏览: 83
好的,下面是两种不同的创建线程的方法:
方法一:使用 Thread 类
```java
public class HelloWorldThread implements Runnable {
public void run() {
for (int i = 0; i < 10; i++) {
System.out.print("Hello");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class WorldThread implements Runnable {
public void run() {
for (int i = 0; i < 10; i++) {
System.out.print("World");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
Thread t1 = new Thread(new HelloWorldThread());
Thread t2 = new Thread(new WorldThread());
t1.start();
t2.start();
t1.join();
t2.join();
}
}
```
方法二:继承 Thread 类
```java
public class HelloWorldThread extends Thread {
public void run() {
for (int i = 0; i < 10; i++) {
System.out.print("Hello");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class WorldThread extends Thread {
public void run() {
for (int i = 0; i < 10; i++) {
System.out.print("World");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) throws InterruptedException {
HelloWorldThread t1 = new HelloWorldThread();
WorldThread t2 = new WorldThread();
t1.start();
t2.start();
t1.join();
t2.join();
}
}
```
这两种方法的输出结果都是每隔 1 秒钟输出一个字符,其中一个输出 "Hello",另一个输出 "World"。
希望我的回答对你有帮助!
阅读全文
相关推荐
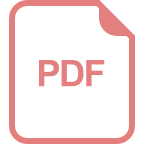
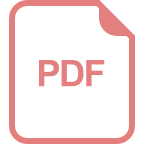
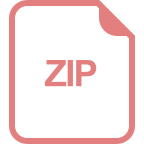
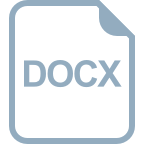
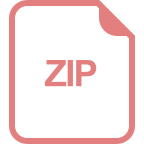
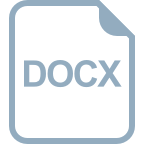
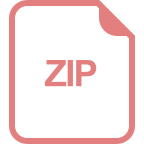
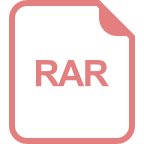
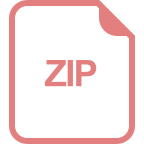
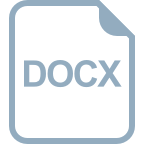