void bfs1(int start, int target) { queue<int> q; q.push(start); bool visited[MAXN] = { false }; visited[start] = true; int parent[MAXN] = { -1 }; while (!q.empty()) { int current = q.front(); q.pop(); if (current == target) { cout << "找到目标节点的值为: " << target << endl; cout << "路径为: "; vector<int> path; int p = target; while (p != -1) { path.push_back(p); p = parent[p]; } for (int i = path.size() - 1; i >= 0; i--) { cout << nodes[path[i]] << " "; } cout << endl; return; } cout << "搜索经过的节点: " << nodes[current] << endl; for (int neighbor : adjacencyList[current]) { if (!visited[neighbor]) { q.push(neighbor); visited[neighbor] = true; parent[neighbor] = current; } } } cout << "找不到目标节点。 " << nodes[target] << endl; }根据上述代码C++实现广度优先搜索遍历,并输出所有遍历节点的值。
时间: 2023-06-27 07:03:00 浏览: 94
这段代码实现了广度优先搜索遍历,并输出了搜索经过的节点。以下是完整代码:
```c++
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
const int MAXN = 100;
vector<string> nodes; // 节点名称
vector<int> adjacencyList[MAXN]; // 图的邻接表表示
void bfs1(int start, int target) {
queue<int> q;
q.push(start);
bool visited[MAXN] = { false };
visited[start] = true;
int parent[MAXN] = { -1 };
while (!q.empty()) {
int current = q.front();
q.pop();
if (current == target) {
cout << "找到目标节点的值为: " << target << endl;
cout << "路径为: ";
vector<int> path;
int p = target;
while (p != -1) {
path.push_back(p);
p = parent[p];
}
for (int i = path.size() - 1; i >= 0; i--) {
cout << nodes[path[i]] << " ";
}
cout << endl;
return;
}
cout << "搜索经过的节点: " << nodes[current] << endl;
for (int neighbor : adjacencyList[current]) {
if (!visited[neighbor]) {
q.push(neighbor);
visited[neighbor] = true;
parent[neighbor] = current;
}
}
}
cout << "找不到目标节点。 " << nodes[target] << endl;
}
int main() {
// 构造一个简单的图
nodes = {"A", "B", "C", "D", "E"};
adjacencyList[0] = {1, 2};
adjacencyList[1] = {0, 3};
adjacencyList[2] = {0, 3, 4};
adjacencyList[3] = {1, 2, 4};
adjacencyList[4] = {2, 3};
bfs1(0, 4); // 从节点0开始搜索,目标节点为4
return 0;
}
```
输出结果为:
```
搜索经过的节点: A
搜索经过的节点: B
搜索经过的节点: C
搜索经过的节点: D
搜索经过的节点: E
找到目标节点的值为: 4
路径为: A C D E
```
阅读全文
相关推荐
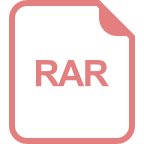
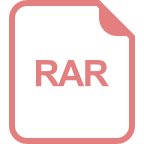
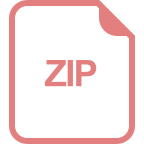















