MOV, MP4格式中封装MJPEG图片的参考代码
时间: 2024-03-10 10:47:14 浏览: 18
以下是使用FFmpeg库将MJPEG格式的图片封装到MOV或MP4文件中的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libavutil/imgutils.h>
int main(int argc, char *argv[]) {
int ret;
AVFormatContext *format_ctx = NULL;
AVStream *video_stream = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVPacket pkt;
AVFrame *frame = NULL;
int width = 640, height = 480;
int fps = 30;
int bitrate = 4000000;
char *filename = "test.mov";
char *image_filename = "image.jpg";
// Initialize FFmpeg
av_register_all();
avcodec_register_all();
// Open output format context
ret = avformat_alloc_output_context2(&format_ctx, NULL, NULL, filename);
if (ret < 0) {
fprintf(stderr, "Failed to allocate output format context: %s\n", av_err2str(ret));
exit(1);
}
// Open video codec
codec = avcodec_find_encoder(format_ctx->oformat->video_codec);
if (!codec) {
fprintf(stderr, "Failed to find video encoder\n");
exit(1);
}
video_stream = avformat_new_stream(format_ctx, NULL);
codec_ctx = avcodec_alloc_context3(codec);
codec_ctx->codec_id = format_ctx->oformat->video_codec;
codec_ctx->width = width;
codec_ctx->height = height;
codec_ctx->pix_fmt = AV_PIX_FMT_YUV420P;
codec_ctx->time_base = (AVRational){1, fps};
codec_ctx->bit_rate = bitrate;
if (format_ctx->oformat->flags & AVFMT_GLOBALHEADER) {
codec_ctx->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
}
ret = avcodec_open2(codec_ctx, codec, NULL);
if (ret < 0) {
fprintf(stderr, "Failed to open video codec: %s\n", av_err2str(ret));
exit(1);
}
avcodec_parameters_from_context(video_stream->codecpar, codec_ctx);
// Open output file
ret = avio_open(&format_ctx->pb, filename, AVIO_FLAG_WRITE);
if (ret < 0) {
fprintf(stderr, "Failed to open output file: %s\n", av_err2str(ret));
exit(1);
}
// Write header
ret = avformat_write_header(format_ctx, NULL);
if (ret < 0) {
fprintf(stderr, "Failed to write header: %s\n", av_err2str(ret));
exit(1);
}
// Encode MJPEG image
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Failed to allocate frame\n");
exit(1);
}
frame->width = width;
frame->height = height;
frame->format = AV_PIX_FMT_YUV420P;
ret = av_frame_get_buffer(frame, 32);
if (ret < 0) {
fprintf(stderr, "Failed to allocate frame buffer: %s\n", av_err2str(ret));
exit(1);
}
AVCodecContext *image_codec_ctx = avcodec_alloc_context3(avcodec_find_decoder(AV_CODEC_ID_MJPEG));
AVStream *image_stream = avformat_new_stream(format_ctx, NULL);
image_stream->id = format_ctx->nb_streams - 1;
avcodec_parameters_from_context(image_stream->codecpar, image_codec_ctx);
avcodec_open2(image_codec_ctx, avcodec_find_decoder(AV_CODEC_ID_MJPEG), NULL);
AVFrame *image_frame = av_frame_alloc();
av_image_fill_arrays(image_frame->data, image_frame->linesize, NULL, AV_PIX_FMT_YUVJ420P, width, height, 1);
AVPacket image_pkt;
av_init_packet(&image_pkt);
ret = avcodec_decode_video2(image_codec_ctx, image_frame, NULL, &image_pkt);
if (ret < 0) {
fprintf(stderr, "Failed to decode image: %s\n", av_err2str(ret));
exit(1);
}
avcodec_free_context(&image_codec_ctx);
av_packet_unref(&image_pkt);
// Encode video frames
for (int i = 0; i < fps; i++) {
// Convert MJPEG image to YUV420P format
struct SwsContext *sws_ctx = sws_getContext(width, height, AV_PIX_FMT_YUVJ420P, width, height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
sws_scale(sws_ctx, image_frame->data, image_frame->linesize, 0, height, frame->data, frame->linesize);
sws_freeContext(sws_ctx);
// Encode video frame
frame->pts = i * (codec_ctx->time_base.den / codec_ctx->time_base.num) / fps;
ret = avcodec_send_frame(codec_ctx, frame);
if (ret < 0) {
fprintf(stderr, "Failed to send frame: %s\n", av_err2str(ret));
exit(1);
}
while (ret >= 0) {
ret = avcodec_receive_packet(codec_ctx, &pkt);
if (ret < 0) {
break;
}
av_packet_rescale_ts(&pkt, codec_ctx->time_base, video_stream->time_base);
pkt.stream_index = video_stream->index;
ret = av_interleaved_write_frame(format_ctx, &pkt);
av_packet_unref(&pkt);
if (ret < 0) {
fprintf(stderr, "Failed to write packet: %s\n", av_err2str(ret));
exit(1);
}
}
}
// Write trailer
ret = av_write_trailer(format_ctx);
if (ret < 0) {
fprintf(stderr, "Failed to write trailer: %s\n", av_err2str(ret));
exit(1);
}
// Cleanup
av_frame_free(&frame);
av_frame_free(&image_frame);
avcodec_free_context(&codec_ctx);
avformat_free_context(format_ctx);
return 0;
}
```
这个示例程序将一个名为`image.jpg`的MJPEG格式的图片封装到一个名为`test.mov`的MOV文件中。你可以根据需要修改代码中的参数,例如视频的分辨率、帧率和码率。注意,这个程序只是一个示例,实际使用时需要根据具体情况进行调整。
相关推荐
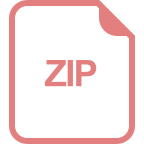














