C# 在方法上标记特性注释,在拦截器中获取
时间: 2024-03-05 11:52:29 浏览: 24
在 C# 中,可以使用特性(Attribute)来标记类、方法、属性等成员,以便在程序运行时通过反射获取这些特性的信息。
为方法标记特性注释,需要定义一个继承自 `System.Attribute` 类的特性类,并将它应用到方法上。比如:
```csharp
[AttributeUsage(AttributeTargets.Method)]
public class MyAttribute : Attribute
{
public string Description { get; set; }
}
public class MyClass
{
[MyAttribute(Description = "This is a method.")]
public void MyMethod()
{
// 方法体
}
}
```
上述代码定义了一个名为 `MyAttribute` 的特性类,并将它应用到 `MyMethod` 方法上。`MyAttribute` 特性类有一个名为 `Description` 的属性,表示方法的描述信息。
在拦截器中获取方法特性注释,可以通过反射获取方法的特性信息。比如:
```csharp
public class MyInterceptor : IInterceptor
{
public void Intercept(IInvocation invocation)
{
var method = invocation.Method;
var attributes = method.GetCustomAttributes(typeof(MyAttribute), true);
if (attributes.Length > 0)
{
var myAttribute = (MyAttribute)attributes[0];
var description = myAttribute.Description;
// 对方法进行拦截处理
}
else
{
// 对方法进行拦截处理
}
}
}
```
上述代码中,`MyInterceptor` 是一个实现了 `Castle.DynamicProxy.IInterceptor` 接口的拦截器类。在拦截方法时,通过 `invocation.Method` 获取当前方法的 `MethodInfo` 对象,然后通过 `GetCustomAttributes` 方法获取该方法上的所有 `MyAttribute` 特性。如果存在 `MyAttribute` 特性,就从中获取 `Description` 属性的值,进行相应的拦截处理。如果不存在该特性,也可以进行默认的拦截处理。
相关推荐
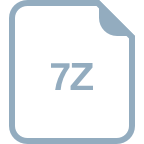














