import numpy as np import pylab as pl import pandas as pd from sklearn.linear_model import Ridge from sklearn.metrics import mean_squared_error from sklearn.model_selection import train_test_split X2=[] X3=[] X4=[] X5=[] X6=[] X7=[] X1=[i for i in range(1,24) for j in range(128)] df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(3,)) X2=df.values.tolist() x2=[] x21=[] for i in X2: if X2.index(i)<=2927: #两个单元楼的分隔数 x2.append(i) else: x21.append(i) df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(4,)) X3=df.values.tolist() x3=[] x31=[] for i in X3: if X3.index(i)<=2927: x3.append(i) else: x31.append(i) df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(5,)) X4=df.values.tolist() x4=[] x41=[] for i in X4: if X4.index(i)<=2927: x4.append(i) else: x41.append(i) df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(6,)) X5=df.values.tolist() x5=[] x51=[] for i in X5: if X5.index(i)<=2927: x5.append(i) else: x51.append(i) df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(7,)) X6=df.values.tolist() x6=[] x61=[] for i in X6: if X6.index(i)<=2927: x6.append(i) else: x61.append(i) df=pd.read_excel('C:/Users/86147/OneDrive/文档/777.xlsx',header=0,usecols=(8,)) X7=df.values.tolist() x7=[] x71=[] for i in X7: if X7.index(i)<=2927: x7.append(i) else: x71.append(i) np.random.seed(42) q=np.array(X1[:2922]) w=np.array(x21[:2922]) e=np.array(x31[:2922]) r=np.array(x41[:2922]) t=np.array(x51[:2922]) p=np.array(x61[:2922]) u=np.array(x71[:2922]) eps=np.random.normal(0,0.05,152) X=np.c_[q,w,e,r,t,p,u] beta=[0.1,0.15,0.2,0.5,0.33,0.45,0.6] y=np.dot(X,beta)X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) alpha = 0.1 # 设置岭回归的惩罚参数 ridge = Ridge(alpha=alpha) ridge.fit(X_train, y_train) y_pred = ridge.predict(X_test) mse = mean_squared_error(y_test, y_pred) print('MSE:', mse) coef = ridge.coef_ # 计算岭回归的系数 intercept = ridge.intercept_ # 计算岭回归的截距 print('Coefficients:', coef) print('Intercept:', intercept)
时间: 2024-04-28 21:21:04 浏览: 240
这段代码是一个基于岭回归的线性回归模型,使用了sklearn库中的Ridge类。它读取一个Excel文件,并将其中的数据用于训练模型。这个模型的目的是预测房价,它使用了七个特征变量(x2到x7)以及一个时间变量(x1)作为输入,预测一个连续的房价输出(y)。该模型使用了训练集和测试集,使用均方误差(MSE)来评估模型的性能。
相关问题
解释如下代码:import numpy as np import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression from sklearn.datasets import make_regression from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler from sklearn.metrics import mean_squared_error
这段代码是导入了一些 Python 的第三方库,其作用如下:
1. `numpy`:Python 数组计算库,提供了高效的数组运算功能。
2. `matplotlib.pyplot`:Python 绘图库,用于绘制图形和数据可视化。
3. `sklearn.linear_model`:Scikit-Learn 库中的线性回归模型。
4. `sklearn.datasets`:Scikit-Learn 库中的数据集生成工具。
5. `sklearn.model_selection`:Scikit-Learn 库中的模型选择工具,用于数据集的分割、交叉验证等操作。
6. `sklearn.preprocessing`:Scikit-Learn 库中的数据预处理工具,用于数据标准化、归一化等操作。
7. `sklearn.metrics`:Scikit-Learn 库中的性能评估工具,用于计算模型的性能指标,如均方误差等。
这些库都是数据分析和机器学习中常用的工具,可以帮助我们更加方便地进行数据处理和模型构建。
解释这段代码import numpy as np import pandas as pd from datetime import datetime from scipy.stats import skew from scipy.special import boxcox1p from scipy.stats import boxcox_normmax from sklearn.linear_model import ElasticNetCV, LassoCV, RidgeCV, Ridge from sklearn.ensemble import GradientBoostingRegressor from sklearn.svm import SVR from sklearn.pipeline import make_pipeline from sklearn.preprocessing import RobustScaler, StandardScaler from sklearn.model_selection import KFold, cross_val_score from sklearn.metrics import mean_squared_error as mse from sklearn.metrics import make_scorer from sklearn.neighbors import LocalOutlierFactor from sklearn.linear_model import LinearRegression from mlxtend.regressor import StackingCVRegressor # from xgboost import XGBRegressor # from lightgbm import LGBMRegressor import matplotlib.pyplot as plt import seaborn as sns
这段代码是在Python中导入所需要的库和模块。具体解释如下:
- `import numpy as np`:导入NumPy库,并将其简写为np,以方便使用。
- `import pandas as pd`:导入Pandas库,并将其简写为pd,以方便使用。
- `from datetime import datetime`:从datetime模块中导入datetime函数,用于处理时间数据。
- `from scipy.stats import skew`:从scipy.stats模块中导入skew函数,用于计算数据的偏度。
- `from scipy.special import boxcox1p`:从scipy.special模块中导入boxcox1p函数,用于进行Box-Cox变换。
- `from scipy.stats import boxcox_normmax`:从scipy.stats模块中导入boxcox_normmax函数,用于计算Box-Cox变换的参数。
- `from sklearn.linear_model import ElasticNetCV, LassoCV, RidgeCV, Ridge`:从sklearn.linear_model模块中导入ElasticNetCV、LassoCV、RidgeCV、Ridge等函数,用于进行线性回归。
- `from sklearn.ensemble import GradientBoostingRegressor`:从sklearn.ensemble模块中导入GradientBoostingRegressor函数,用于进行梯度提升回归。
- `from sklearn.svm import SVR`:从sklearn.svm模块中导入SVR函数,用于进行支持向量回归。
- `from sklearn.pipeline import make_pipeline`:从sklearn.pipeline模块中导入make_pipeline函数,用于构建机器学习管道。
- `from sklearn.preprocessing import RobustScaler, StandardScaler`:从sklearn.preprocessing模块中导入RobustScaler、StandardScaler函数,用于进行特征缩放。
- `from sklearn.model_selection import KFold, cross_val_score`:从sklearn.model_selection模块中导入KFold、cross_val_score函数,用于进行交叉验证。
- `from sklearn.metrics import mean_squared_error as mse`:从sklearn.metrics模块中导入mean_squared_error函数,并将其简写为mse,用于计算均方误差。
- `from sklearn.metrics import make_scorer`:从sklearn.metrics模块中导入make_scorer函数,用于创建自定义评分函数。
- `from sklearn.neighbors import LocalOutlierFactor`:从sklearn.neighbors模块中导入LocalOutlierFactor函数,用于检测异常值。
- `from sklearn.linear_model import LinearRegression`:从sklearn.linear_model模块中导入LinearRegression函数,用于进行线性回归。
- `from mlxtend.regressor import StackingCVRegressor`:从mlxtend.regressor模块中导入StackingCVRegressor函数,用于进行交叉验证的堆叠模型。
- `import matplotlib.pyplot as plt`:导入matplotlib库,并将其简写为plt,用于绘制图形。
- `import seaborn as sns`:导入seaborn库,并将其简写为sns,用于绘制图形。
阅读全文
相关推荐
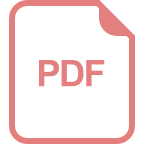
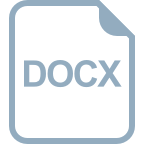
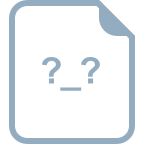














