plt.plot(history.history['loss'],name='Model Loss') plt.plot(history.history['val_loss'],name='Model Accuracy')raise AttributeError( AttributeError: Line2D.set() got an unexpected keyword argument 'name'
时间: 2023-08-05 09:01:53 浏览: 47
这个错误是因为在 `plt.plot()` 中使用了不支持的参数 `name`,应该使用 `label` 参数来设置标签。您可以将代码改为以下形式:
```
plt.plot(history.history['loss'], label='Model Loss')
plt.plot(history.history['val_loss'], label='Model Accuracy')
plt.legend()
```
这样就可以给每个线条设置标签,并使用 `legend()` 函数来显示图例。
相关问题
plt.plot(history.history['loss'],label='train_loss') plt.plot(history.history['val_loss'],label='val_loss')为什么不显示曲线标签
这个问题可能是因为你没有使用plt.legend()命令来显示标签。在plt.plot()命令中使用label参数来指定曲线的标签是正确的,但是还需要使用plt.legend()来显示这些标签。请尝试在这两个plt.plot()命令之后加上plt.legend()命令,如下所示:
```
plt.plot(history.history['loss'],label='train_loss')
plt.plot(history.history['val_loss'],label='val_loss')
plt.legend()
```
这应该会显示出曲线标签。如果仍然没有显示,请检查是否有其他代码覆盖了这个部分的设置。
plt.plot(history.epoch, history.history.get('loss'), label='loss') plt.plot(history.epoch, history.history.get('val_loss'), label='val_loss') plt.legend()
这段代码使用matplotlib库绘制了训练损失(loss)和验证损失(val_loss)随时间的变化曲线。
`plt.plot(history.epoch, history.history.get('loss'), label='loss')`绘制了训练损失随时间的变化曲线。`history.epoch`表示训练的迭代次数,`history.history.get('loss')`获取了训练过程中记录的损失值的数值。`label='loss'`指定了曲线的标签为'loss'。
同样地,`plt.plot(history.epoch, history.history.get('val_loss'), label='val_loss')`绘制了验证损失随时间的变化曲线。
最后,通过调用`plt.legend()`方法添加图例,以便区分训练损失和验证损失的曲线。
这段代码用于可视化训练过程中损失的变化情况,可以帮助我们分析模型的训练效果和过拟合情况。
相关推荐
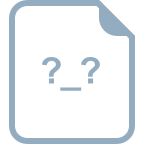
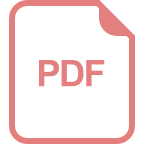
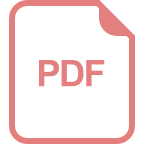












