pycharm中发送带有图片的邮件
时间: 2024-11-10 11:13:46 浏览: 5
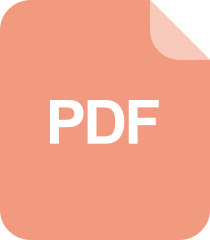
pycharm中成功运行图片的配置教程
在PyCharm中发送带有图片的邮件通常涉及到SMTP库的使用,以及处理附件。以下是一个简单的步骤指南:
1. 安装必要的库:首先确保安装了`smtplib`和`email`库,如果还没有安装,可以在命令行中运行 `pip install smtplib email`
2. 导入库并设置SMTP服务器信息:
```python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
```
3. 创建邮件内容:
- 创建一个主邮件对象(MIMEMultipart)
- 添加文本部分(MIMEText)
- 添加图片作为附件:
```python
with open('image_path.jpg', 'rb') as img_file:
image = MIMEBase('image', 'jpg')
image.set_payload(img_file.read())
encoders.encode_base64(image)
image.add_header('Content-Disposition', f'attachment; filename="{img_file.name}"')
```
4. 设置发件人、收件人、主题等信息:
```python
msg = MIMEMultipart()
msg['From'] = 'your_email@example.com'
msg['To'] = 'recipient_email@example.com'
msg['Subject'] = 'Email with Image'
text = MIMEText('这是包含图片的消息')
msg.attach(text)
msg.attach(image)
```
5. 连接到SMTP服务器,发送邮件:
```python
smtp_server = 'smtp.example.com'
smtp_port = 587
username = 'your_username'
password = 'your_password'
with smtplib.SMTP(smtp_server, smtp_port) as server:
server.starttls() # 如果需要加密连接
server.login(username, password)
server.send_message(msg)
```
记得替换上述示例中的`image_path`, `smtp_server`, `smtp_port`, `username`, 和 `password`为实际值。
阅读全文
相关推荐
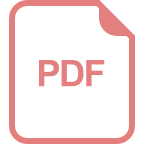

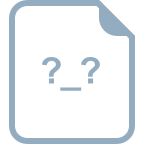
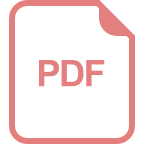
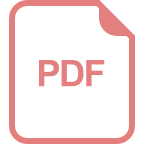
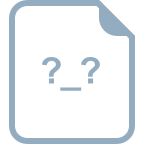
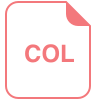
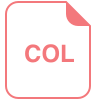
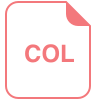
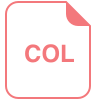
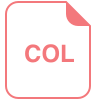
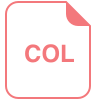
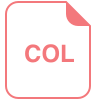
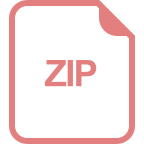
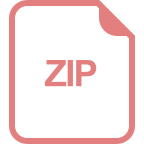
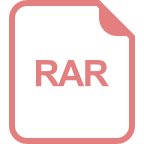
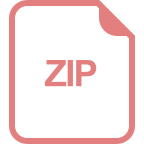