用Java实现权限管理
时间: 2023-09-26 21:05:59 浏览: 48
在Java中实现权限管理有多种方式,这里提供一种基于RBAC(Role-Based Access Control)模型的实现方法,具体步骤如下:
1. 定义角色和权限
首先需要定义系统中的角色和权限,可以使用数据库表来存储角色和权限信息,例如创建角色表和权限表:
```
CREATE TABLE role (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
PRIMARY KEY (id)
);
CREATE TABLE permission (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
url VARCHAR(200) NOT NULL,
PRIMARY KEY (id)
);
```
2. 建立角色和权限的关系
接下来需要建立角色和权限之间的关系,可以使用数据库表来存储角色和权限之间的对应关系,例如创建角色权限表:
```
CREATE TABLE role_permission (
id INT(11) NOT NULL AUTO_INCREMENT,
role_id INT(11) NOT NULL,
permission_id INT(11) NOT NULL,
PRIMARY KEY (id),
FOREIGN KEY (role_id) REFERENCES role(id),
FOREIGN KEY (permission_id) REFERENCES permission(id)
);
```
3. 实现用户登录和授权
当用户登录系统时,需要根据用户的角色查询其拥有的权限,然后保存到用户的会话中,以便后续的访问中进行权限判断。可以使用Java的Web框架来实现用户登录和授权的功能,例如使用Spring Security框架实现:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.defaultSuccessUrl("/")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在上述代码中,使用了`@EnableWebSecurity`注解启用了Spring Security功能,通过重写`configure(HttpSecurity http)`方法来定义访问规则和登录页面等设置,使用`@Autowired`注解注入`UserDetailsService`对象,并通过重写`configure(AuthenticationManagerBuilder auth)`方法来配置用户认证方式。
4. 实现RBAC权限控制
最后需要实现基于RBAC模型的权限控制,可以在代码中添加如下方法:
```
@Autowired
private RoleService roleService;
@Autowired
private PermissionService permissionService;
public boolean hasPermission(HttpServletRequest request, Authentication authentication) {
Object principal = authentication.getPrincipal();
if (principal instanceof UserDetails) {
String username = ((UserDetails) principal).getUsername();
List<Role> roles = roleService.getRolesByUser(username);
for (Role role : roles) {
List<Permission> permissions = permissionService.getPermissionsByRole(role.getName());
for (Permission permission : permissions) {
AntPathMatcher antPathMatcher = new AntPathMatcher();
if (antPathMatcher.match(permission.getUrl(), request.getRequestURI())) {
return true;
}
}
}
}
return false;
}
```
该方法首先获取当前登录用户的用户名,然后根据用户名查询其拥有的角色,进而查询角色对应的权限列表,最后使用AntPathMatcher类来进行URL匹配,如果匹配成功则返回true,否则返回false。
在需要进行权限控制的方法中,可以通过注解的方式来调用上述方法,例如:
```
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private SecurityService securityService;
@GetMapping("/profile")
@PreAuthorize("@securityService.hasPermission(request, authentication)")
public ResponseEntity<?> getProfile(HttpServletRequest request, Authentication authentication) {
// TODO: 实现获取用户个人信息的功能
}
}
```
在上述代码中,使用了`@PreAuthorize("@securityService.hasPermission(request, authentication)")`注解来指定调用`hasPermission()`方法进行权限判断。
相关推荐
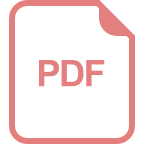
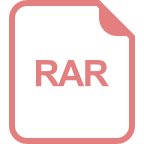
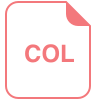
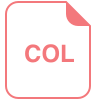
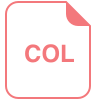
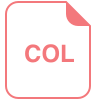
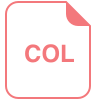









