java长连接的实现方式,附上代码实现
时间: 2024-06-09 15:07:34 浏览: 112
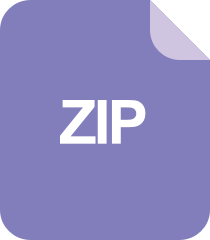
java socket 长连接实例

Java长连接的实现方式通常有两种:基于Socket和基于Netty框架。下面简单介绍一下这两种方式的实现方法,并提供相关的代码示例供参考。
1. 基于Socket实现长连接
基于Socket实现长连接的方法比较简单,通过在客户端和服务器端建立Socket连接,并不断发送和接收数据来保持连接。具体实现方法如下:
客户端代码示例:
```java
public class SocketClient {
private static final String SERVER_IP = "127.0.0.1";
private static final int SERVER_PORT = 8888;
public static void main(String[] args) {
try {
Socket socket = new Socket(SERVER_IP, SERVER_PORT);
while (true) {
// 发送数据
OutputStream outputStream = socket.getOutputStream();
String message = "Hello, Server!";
outputStream.write(message.getBytes("UTF-8"));
outputStream.flush();
System.out.println("Send message to server: " + message);
// 接收数据
InputStream inputStream = socket.getInputStream();
byte[] data = new byte[1024];
int len = inputStream.read(data);
String response = new String(data, 0, len, "UTF-8");
System.out.println("Receive message from server: " + response);
// 暂停1秒钟
Thread.sleep(1000);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
服务器端代码示例:
```java
public class SocketServer {
private static final int SERVER_PORT = 8888;
public static void main(String[] args) {
try {
ServerSocket serverSocket = new ServerSocket(SERVER_PORT);
Socket socket = serverSocket.accept();
System.out.println("Client connected!");
while (true) {
// 接收数据
InputStream inputStream = socket.getInputStream();
byte[] data = new byte[1024];
int len = inputStream.read(data);
String message = new String(data, 0, len, "UTF-8");
System.out.println("Receive message from client: " + message);
// 发送数据
OutputStream outputStream = socket.getOutputStream();
String response = "Hello, Client!";
outputStream.write(response.getBytes("UTF-8"));
outputStream.flush();
System.out.println("Send message to client: " + response);
// 暂停1秒钟
Thread.sleep(1000);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
2. 基于Netty框架实现长连接
Netty框架是一个高性能、异步事件驱动的网络编程框架,可以方便地实现长连接。具体实现方法如下:
客户端代码示例:
```java
public class NettyClient {
private static final String SERVER_IP = "127.0.0.1";
private static final int SERVER_PORT = 8888;
public static void main(String[] args) {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap()
.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringEncoder());
pipeline.addLast(new StringDecoder());
pipeline.addLast(new NettyClientHandler());
}
});
ChannelFuture future = bootstrap.connect(SERVER_IP, SERVER_PORT).sync();
System.out.println("Client connected!");
while (true) {
// 发送数据
Channel channel = future.channel();
String message = "Hello, Server!";
channel.writeAndFlush(message);
System.out.println("Send message to server: " + message);
// 暂停1秒钟
Thread.sleep(1000);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
group.shutdownGracefully();
}
}
}
```
服务器端代码示例:
```java
public class NettyServer {
private static final int SERVER_PORT = 8888;
public static void main(String[] args) {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new StringEncoder());
pipeline.addLast(new StringDecoder());
pipeline.addLast(new NettyServerHandler());
}
});
ChannelFuture future = bootstrap.bind(SERVER_PORT).sync();
System.out.println("Server started!");
future.channel().closeFuture().sync();
} catch (Exception e) {
e.printStackTrace();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
}
```
需要注意的是,Netty框架中的数据传输是以ByteBuf对象为单位进行的,因此需要在ChannelPipeline中添加相应的编码器和解码器,将字符串转换为ByteBuf对象进行传输。另外,Netty框架中的ChannelHandler可以监听各种事件,可以方便地实现长连接的心跳检测等功能。具体实现可参考以下示例代码:
NettyClientHandler.java:
```java
public class NettyClientHandler extends SimpleChannelInboundHandler<String> {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
System.out.println("Channel active!");
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
System.out.println("Channel inactive!");
ctx.channel().close();
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Receive message from server: " + msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.channel().close();
}
}
```
NettyServerHandler.java:
```java
public class NettyServerHandler extends SimpleChannelInboundHandler<String> {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
System.out.println("Channel active!");
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
System.out.println("Channel inactive!");
ctx.channel().close();
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
System.out.println("Receive message from client: " + msg);
// 发送数据
String response = "Hello, Client!";
ctx.writeAndFlush(response);
System.out.println("Send message to client: " + response);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
cause.printStackTrace();
ctx.channel().close();
}
}
```
阅读全文
相关推荐
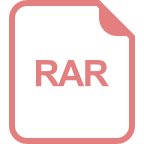

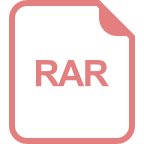
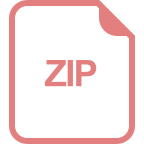
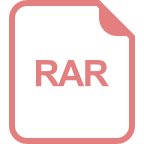
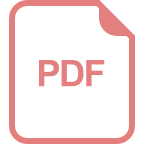
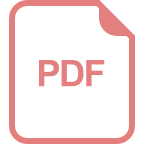
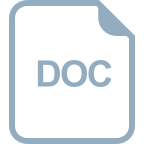
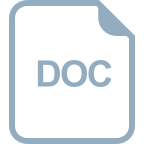
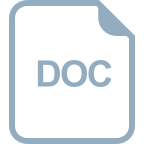
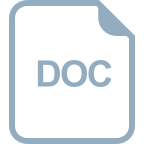
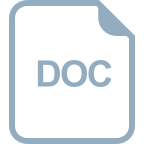
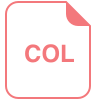
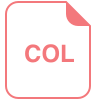

