const express = require('express') const cors = require('cors') const path = require('path') const app = express() app.use(cors({ origin:'*' })) app.get('/', (req, res) => res.send('Hello World!')) app.use(express.static(path.join(__dirname, 'www'))) app.listen(3000, () => console.log('Example app listening on port 3000!'))
时间: 2024-01-14 22:02:48 浏览: 25
这段代码使用了Node.js的Express框架创建了一个Web应用程序,并启用了CORS支持。它还使用了静态文件中间件来提供静态文件服务,例如HTML、CSS、JavaScript和图像文件。最后,它将服务器监听在3000端口上,以便在浏览器中访问应用程序。当用户访问根路由时,它将返回“Hello World!”文本。
相关问题
const express = require('express') const cors = require('cors') const path = require('path') const app = express() app.use(cors({ origin:'*' })) app.get('/', (req, res) => res.send('Hello World!')) app.use(express.static(path.join(__dirname, 'www'))) app.listen(3000, () => console.log('Example app listening on port 3000!'))在这个代码里改
可以将代码修改如下:
```javascript
const express = require('express');
const cors = require('cors');
const path = require('path');
const app = express();
app.use(cors({ origin:'*' }));
app.get('/', (req, res) => res.send('Hello World!'));
app.use(express.static(path.join(__dirname, 'www')));
app.get('/api/data', (req, res) => {
res.setHeader('Content-Type', 'application/json');
res.setHeader('Access-Control-Allow-Origin', '*');
const data = { message: '这是一个跨域请求的响应数据!' };
res.send(JSON.stringify(data));
});
app.listen(3000, () => console.log('Example app listening on port 3000!'));
```
这里新增了一个路由`/api/data`,用于返回一个包含`message`属性的JSON数据。同时在响应头中添加`Content-Type`和`Access-Control-Allow-Origin`,以便允许跨域请求访问该接口。
你可以将这段代码保存为`app.js`文件,然后在终端中执行`node app.js`命令来启动服务器,在浏览器中访问`http://localhost:3000/api/data`,就可以看到返回的JSON数据了。
const cors = require('cors')是什么意思
`const cors = require('cors')` 表示在 Node.js 应用程序中引入了 cors 中间件。cors (Cross-Origin Resource Sharing) 是一种 Web 应用程序安全机制,用于控制跨域资源共享。通过使用 cors 中间件,你可以解决浏览器的同源策略限制,从而允许跨域请求资源。
在上述代码中,我们使用 `require()` 函数将 cors 模块导入到文件中,然后将其分配给名为 `cors` 的常量。然后,我们使用 `app.use()` 函数将 cors 中间件添加到 Express 应用程序中,以便在处理 HTTP 请求时控制跨域资源共享。这样,我们就可以在不受浏览器的同源策略限制的情况下向其他域名或端口请求资源。
相关推荐
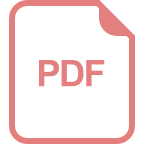
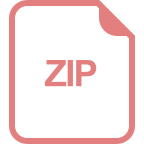
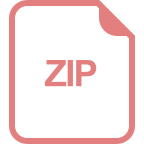













