写一个能够实现自我学习的ai程序
时间: 2023-11-18 18:04:22 浏览: 64
要实现自我学习的 AI 程序,需要使用机器学习算法和技术。这里给出一个简单的示例,使用 C# 和 Microsoft Azure 的自然语言处理服务来实现一个能够学习的聊天机器人。
首先,你需要注册一个 Azure 帐号并启用自然语言处理服务。然后,你需要安装 Azure SDK 和 NuGet 包管理器。接下来,你可以创建一个 C# 控制台应用程序,并将以下代码添加到 Program.cs 文件中:
```
using System;
using System.Threading.Tasks;
using Microsoft.Azure.CognitiveServices.Language.LUIS.Runtime;
using Microsoft.Azure.CognitiveServices.Language.LUIS.Runtime.Models;
namespace SelfLearningAI
{
class Program
{
static async Task Main(string[] args)
{
Console.WriteLine("Hello, I am a self-learning AI. How can I assist you today?");
var appId = "your_LUIS_app_id";
var subscriptionKey = "your_LUIS_subscription_key";
var endpoint = "https://your_LUIS_endpoint.api.cognitive.microsoft.com";
var credentials = new ApiKeyServiceClientCredentials(subscriptionKey);
var client = new LUISRuntimeClient(credentials) { Endpoint = endpoint };
while (true)
{
var input = Console.ReadLine();
var prediction = await client.Prediction.ResolveAsync(appId, input);
if (prediction.TopIntent().Intent == "Greeting")
{
Console.WriteLine("Hello there!");
}
else if (prediction.TopIntent().Intent == "Goodbye")
{
Console.WriteLine("Goodbye!");
break;
}
else
{
Console.WriteLine("I'm sorry, I don't understand. Can you please try again?");
}
// Train the model with the user's input
var userInput = new Utterance
{
Text = input,
IntentName = prediction.TopIntent().Intent,
Entities = prediction.Entities,
};
await client.Train.TrainVersionAsync(appId, 0, new[] { userInput });
await client.Train.PublishAppAsync(appId, isStaging: true);
}
}
}
}
```
在这个程序中,我们使用了 Microsoft Azure 的 LUIS 自然语言处理服务来识别用户的意图和实体。每当用户输入一条消息时,我们会调用 LUIS 的预测 API 来获取用户的意图和实体。然后,我们可以根据意图来回答用户的问题,并将用户的输入和意图保存到 LUIS 模型中以进行训练。通过不断地训练,我们的 AI 程序可以变得越来越智能,能够识别更多的意图和实体。
需要注意的是,这只是一个简单的示例,真正的自我学习 AI 程序需要更复杂的算法和技术,涉及到深度学习、增强学习等领域。如果你想进一步了解自我学习 AI 的实现原理和技术,可以参考相关的机器学习和人工智能教程。
相关推荐
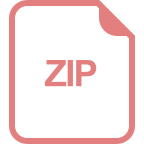
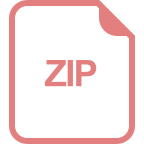
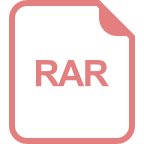














