类fact中包含数据成员类型有整型数据n,m,其中m的值为n的阶乘;公有成员函数包括:1)包含默认参数的构造函数,n的默认值为0;2)将n值加1的函数;3)计算m值的函数4)对<<运算符进行友元重载,输出格式为“3!=6”。要求编写main函数使用fact类的对象输出1-20的阶乘
时间: 2023-06-10 20:06:38 浏览: 61
以下是fact类的代码实现:
```c++
#include <iostream>
using namespace std;
class fact {
private:
int n;
int m;
public:
fact(int num = 0) {
n = num;
m = 1;
for (int i = 1; i <= n; i++) {
m *= i;
}
}
void addOne() {
n++;
m *= n;
}
int getM() {
return m;
}
friend ostream& operator<<(ostream& os, const fact& f) {
os << f.n << "! = " << f.m;
return os;
}
};
int main() {
for (int i = 1; i <= 20; i++) {
fact f(i);
cout << f << endl;
}
return 0;
}
```
在main函数中,我们通过循环从1到20,创建了每个数的阶乘对象,并输出其结果。
相关问题
用c语言类fact中包含数据成员类型有整型数据n,m,其中m的值为n的阶乘;公有成员函数包括:1)包含默认参数的构造函数,n的默认值为0;2)将n值加1的函数;3)计算m值的函数4)对<<运算符进行友元重载,输出格式为“3!=6”。要求编写main函数使用fact类的对象输出1-20的阶乘
好的,我明白了。以下是代码实现:
```
#include <iostream>
using namespace std;
class fact {
private:
int n;
int m;
public:
fact(int num = 0) {
n = num;
m = calculateM();
}
void addN() {
n++;
m = calculateM();
}
int calculateM() {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
friend ostream& operator<<(ostream& os, const fact& f) {
os << f.n << "! = " << f.m;
return os;
}
};
int main() {
for (int i = 1; i <= 20; i++) {
fact f(i);
cout << f << endl;
}
return 0;
}
```
以上代码中,我们定义了一个名为 fact 的类,其中包含数据成员 n 和 m,分别表示阶乘的计算数和结果。我们还定义了四个公有函数,包括构造函数、将 n 值加 1 的函数、计算 m 值的函数和友元重载运算符<< 用于输出结果。最后,我们在 main 函数中使用循环输出 1-20 的阶乘结果,具体实现过程中,我们利用对象 f 第一次调用 calculateM() 函数时便得到了阶乘结果,从而避免类中数据成员值改变时重新计算阶乘所花费的时间。
(1)定义函数fact(n)计算n的阶乘:函数的返回值类型是double型
函数定义如下:
```c
double fact(int n) {
double result = 1.0;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
```
函数解释:
- 参数:整型n,表示要计算阶乘的数。
- 返回值:双精度浮点型(double),表示n的阶乘。
- 函数实现:使用循环计算n的阶乘,将每次循环得到的结果乘到result中,最后返回result即可。
相关推荐
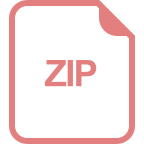
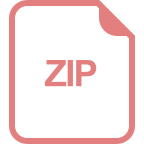












