import requests from bs4 import BeautifulSoup import re import json def getKeywordResult(keyword): url = 'http://www.baidu.com/s?wd='+keyword try: r = requests.get(url, timeout=30) r.raise_for_status() r.encoding = 'utf-8' return r.text except: return "" def parserLinks(html): soup = BeautifulSoup(html, "html.parser") links = [] for div in soup.find_all('div', {'data-tools': re.compile('title')}): data = div.attrs['data-tools'] #获得属性值 d = json.loads(data) #将属性值转换成字典 links.append(d['title']) #将返回链接的题目返回 return links def main(): html = getKeywordResult('Python语言程序设计基础(第2版)') ls = parserLinks(html) count = 1 for i in ls: print("[{:^3}]{}".format(count, i)) count += 1 main()
时间: 2024-04-27 22:19:23 浏览: 16
这段代码的作用是在百度搜索中搜索关键词"Python语言程序设计基础(第2版)",然后解析搜索结果页面中的链接和标题,并将这些标题列成一个列表进行输出。
具体来说,这段代码使用了 requests 库向百度搜索发送了一个 GET 请求,并将返回的页面内容传递给了 parserLinks 函数进行解析。解析函数使用了 BeautifulSoup 库来解析 HTML 页面,并使用正则表达式和 JSON 库来获取页面中的链接和标题信息。最后,这些标题被按照一定的格式输出到标准输出流中。
如果您想了解更多关于 requests、BeautifulSoup、正则表达式和 JSON 库的信息,建议您查阅相关文档或者学习相关的课程。
相关问题
以下代码爬取的内容是乱码,什么原因?from bs4 import BeautifulSoup import requests if name == 'main': url = 'https://www.pincai.com/article/2320333.htm' response = requests.get(url).text soup = BeautifulSoup(response, 'lxml')。帮我修改好代码
以下是修改后的代码:
```python
from bs4 import BeautifulSoup
import requests
if __name__ == '__main__':
url = 'https://www.pincai.com/article/2320333.htm'
response = requests.get(url)
response.encoding = 'utf-8'
soup = BeautifulSoup(response.text, 'lxml')
# 在这里可以继续处理网页内容
```
对于乱码问题,我在代码中添加了 `response.encoding = 'utf-8'` 来指定网页的编码为UTF-8,以确保正确解析网页内容。另外,我将 `response.text` 传递给 `BeautifulSoup` 来处理,以获取正确的解析结果。
现在你可以继续处理 `soup` 对象中的网页内容了。如果还有其他问题,请随时提问。
import requests from bs4 import BeautifulSoup import re import docx from docx.oxml.ns import qn
这段代码缺少必要的语法结构。请将这些语句放置在一个函数或方法中,例如:
```python
import requests
from bs4 import BeautifulSoup
import re
import docx
from docx.oxml.ns import qn
def my_function():
# 在这里编写代码
```
请注意,这只是一个示例,您需要根据您的实际需求来编写函数或方法。在您的函数或方法中,您可以使用上述导入的库和模块来完成您的任务。
相关推荐
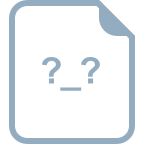
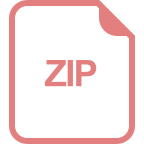












