用c语言代码输出无向带权图的邻接矩阵和邻接表
时间: 2024-04-29 22:27:09 浏览: 127
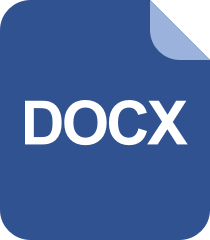
数据结构学习--图的邻接矩阵和邻接表存储
可以的,以下是用C语言代码输出无向带权图的邻接矩阵和邻接表的程序:
```
#include <stdio.h>
#define MAX_VERTICES 100
typedef struct {
int edges[MAX_VERTICES][MAX_VERTICES]; // 邻接矩阵
int degree[MAX_VERTICES]; // 存储每个节点的度
int nvertices; // 节点数
} Graph;
void init_graph(Graph* graph, int n)
{
graph->nvertices = n;
for (int i = 0; i < n; i++) {
graph->degree[i] = 0;
for (int j = 0; j < n; j++) {
graph->edges[i][j] = 0; // 初始化所有图中边的权重为0
}
}
}
void add_edge(Graph* graph, int u, int v, int w)
{
graph->edges[u][v] = w; // 设置边的权重
graph->edges[v][u] = w; // 无向图,两个方向都要设置
graph->degree[u]++; // 节点u的度+1
graph->degree[v]++; // 节点v的度+1
}
void print_matrix(Graph* graph)
{
printf("邻接矩阵:\n");
for (int i = 0; i < graph->nvertices; i++) {
for (int j = 0; j < graph->nvertices; j++) {
printf("%2d ", graph->edges[i][j]);
}
printf("\n");
}
}
void print_list(Graph* graph)
{
printf("邻接表:\n");
for (int i = 0; i < graph->nvertices; i++) {
printf("%d -> ", i);
for (int j = 0; j < graph->nvertices; j++) {
if (graph->edges[i][j] > 0) {
printf("%d(%d) ", j, graph->edges[i][j]);
}
}
printf("\n");
}
}
int main()
{
Graph graph;
int n = 6;
init_graph(&graph, n);
add_edge(&graph, 0, 1, 2);
add_edge(&graph, 0, 2, 3);
add_edge(&graph, 1, 3, 1);
add_edge(&graph, 1, 4, 4);
add_edge(&graph, 2, 4, 5);
add_edge(&graph, 3, 5, 6);
add_edge(&graph, 4, 5, 7);
print_matrix(&graph);
print_list(&graph);
return 0;
}
```
在上面的程序中,我们定义了一个 Graph 结构体,用于存储无向带权图的邻接矩阵和邻接表。在初始化图时,我们将节点数 n 作为参数传入 init_graph 函数中,然后将图中每个边的权重都初始化为0,节点的度初始化为0。在添加边时,我们需要传入两个节点的编号和边的权重,然后将对应的两个节点的度+1,同时将对应的两个节点之间的边的权重设置为我们传入的值。最后,我们可以分别使用 print_matrix 和 print_list 函数输出图的邻接矩阵和邻接表。
如果你有任何问题,欢迎继续向我提问。
阅读全文
相关推荐
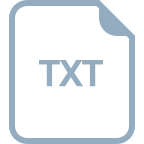
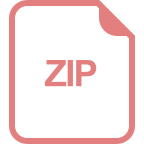














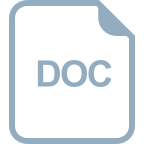
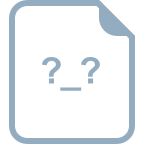