Given 3 points on a 2D plane, design a function to do the following: determine whether 3 points lie on a straight line; if they do not lie on a straight line, draw a triangle with those points. (please import matplotlib) Note that the straight line or one of the triangle's edges may be perpendicular to the x-axis. Please take this possibility into account. The following shows the execution of your program. n=[[1,1],[2,2],[3,3]] threepoints(n) #output They lie on a straight line. ---------------------------------------------------------------------------- m=[[1,1],[2,3],[4,2]] threepoints(m) #output
时间: 2023-08-15 17:10:39 浏览: 177
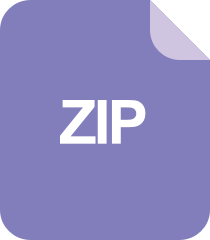
points-3d-to-2d:将3D点投影到2D平面上
They form a triangle.
Here is the code to implement this:
```python
import matplotlib.pyplot as plt
def threepoints(points):
# extract coordinates of the three points
x1, y1 = points[0]
x2, y2 = points[1]
x3, y3 = points[2]
# check if the three points are collinear
if (y2-y1)*(x3-x2) == (y3-y2)*(x2-x1):
print("They lie on a straight line.")
# plot the points on a graph
plt.plot([x1, x2, x3], [y1, y2, y3], 'o')
plt.show()
else:
print("They form a triangle.")
# plot the points and connect them to form a triangle
plt.plot([x1, x2, x3, x1], [y1, y2, y3, y1], '-')
plt.show()
```
The function first extracts the x and y coordinates of the three points provided in the input list. It then checks if the three points are collinear by calculating the slope of the line passing through the first two points and comparing it to the slope of the line passing through the second and third points. If the slopes are equal, then the points are collinear and the function prints a message indicating so and plots the points on a graph. If the slopes are not equal, then the function prints a message indicating that the points form a triangle and plots the points connected by lines to form a triangle.
阅读全文
相关推荐
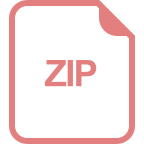









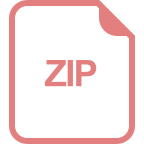
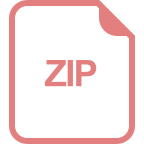
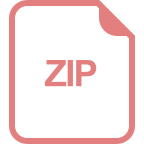
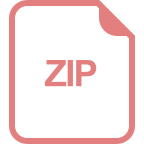
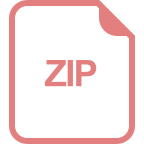
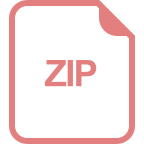
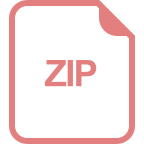