用java语言编写一个建造器模式
时间: 2023-04-03 20:04:07 浏览: 63
建造器模式是一种创建型设计模式,它允许您创建不同类型的对象,而无需暴露对象的创建逻辑。在Java中,您可以使用以下代码编写建造器模式:
public class House {
private String foundation;
private String structure;
private String roof;
private String interior;
public House(HouseBuilder builder) {
this.foundation = builder.foundation;
this.structure = builder.structure;
this.roof = builder.roof;
this.interior = builder.interior;
}
public static class HouseBuilder {
private String foundation;
private String structure;
private String roof;
private String interior;
public HouseBuilder() {}
public HouseBuilder foundation(String foundation) {
this.foundation = foundation;
return this;
}
public HouseBuilder structure(String structure) {
this.structure = structure;
return this;
}
public HouseBuilder roof(String roof) {
this.roof = roof;
return this;
}
public HouseBuilder interior(String interior) {
this.interior = interior;
return this;
}
public House build() {
return new House(this);
}
}
}
在上面的代码中,我们定义了一个House类和一个HouseBuilder类。House类具有四个属性:foundation、structure、roof和interior。HouseBuilder类具有与House类相同的四个属性,并且还有四个方法,用于设置这些属性。最后,我们在House类中定义了一个构造函数,该构造函数接受一个HouseBuilder对象,并使用它来设置House对象的属性。我们还定义了一个build()方法,该方法返回一个新的House对象。
相关推荐
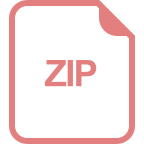
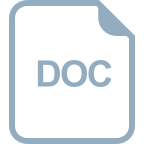
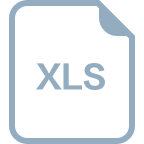














