java写GUI界面实现文件拷贝
时间: 2024-03-10 18:50:26 浏览: 93
好的,您可以使用Java自带的Swing框架来实现一个简单的GUI界面,然后使用Java IO库来完成文件拷贝的功能。
以下是一个简单的实现示例:
```java
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import javax.swing.*;
public class CopyFileGUI {
private JFrame frame;
private JButton srcButton;
private JButton destButton;
private JButton copyButton;
private JLabel statusLabel;
private String srcPath;
private String destPath;
public CopyFileGUI() {
// 创建GUI界面
frame = new JFrame("文件拷贝");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 150);
frame.setLayout(new GridLayout(4, 1));
// 创建选择源文件按钮
srcButton = new JButton("选择源文件");
srcButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser chooser = new JFileChooser();
int returnVal = chooser.showOpenDialog(frame);
if (returnVal == JFileChooser.APPROVE_OPTION) {
srcPath = chooser.getSelectedFile().getAbsolutePath();
System.out.println(srcPath);
}
}
});
frame.add(srcButton);
// 创建选择目标文件夹按钮
destButton = new JButton("选择目标文件夹");
destButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JFileChooser chooser = new JFileChooser();
chooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int returnVal = chooser.showOpenDialog(frame);
if (returnVal == JFileChooser.APPROVE_OPTION) {
destPath = chooser.getSelectedFile().getAbsolutePath();
System.out.println(destPath);
}
}
});
frame.add(destButton);
// 创建拷贝按钮
copyButton = new JButton("开始拷贝");
copyButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (srcPath == null || srcPath.isEmpty()) {
statusLabel.setText("请选择源文件!");
return;
}
if (destPath == null || destPath.isEmpty()) {
statusLabel.setText("请选择目标文件夹!");
return;
}
try {
// 拷贝文件
FileInputStream inputStream = new FileInputStream(srcPath);
FileOutputStream outputStream = new FileOutputStream(destPath + File.separator + new File(srcPath).getName());
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
inputStream.close();
outputStream.close();
statusLabel.setText("文件拷贝成功!");
} catch (IOException ex) {
statusLabel.setText("文件拷贝失败:" + ex.getMessage());
}
}
});
frame.add(copyButton);
// 创建状态标签
statusLabel = new JLabel("", JLabel.CENTER);
frame.add(statusLabel);
// 显示GUI界面
frame.setVisible(true);
}
public static void main(String[] args) {
new CopyFileGUI();
}
}
```
运行以上代码,即可看到一个简单的文件拷贝GUI界面。
阅读全文
相关推荐
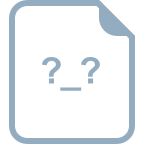

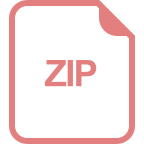



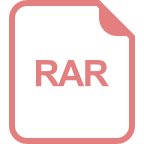
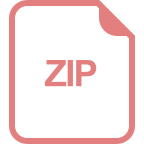
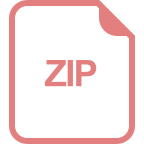
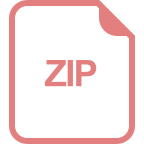
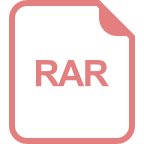
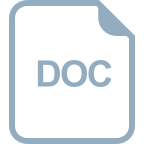
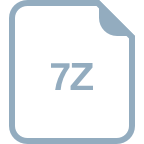
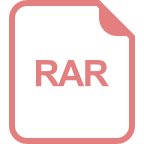
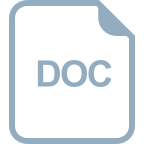
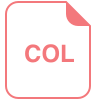
