#include <stdio.h> #include <string.h> // 定义结构体类型 struct Student { char id[10]; // 学号 char name[20]; // 姓名 char gender[10]; // 性别 int chinese; // 语文成绩 int math; // 数学成绩 int english; // 英语成绩 }; // 初始化几个学生的基本信息 struct Student students[] = { {"1001", "张三", "Male", 80, 90, 85}, {"1002", "李四", "Female", 75, 85, 90}, {"1003", "王五", "Male", 90, 80, 95}, {"1004", "赵六", "Female", 85, 95, 80}, {"1005", "钱七", "Male", 95, 85, 90} };接着写一个函数,要求在不新建数组的情况下实现添加一个学生
时间: 2023-07-19 16:18:39 浏览: 46
可以通过动态分配内存的方式来实现添加一个学生,具体实现如下:
```c
// 添加学生的函数,参数为一个学生的结构体指针
void add_student(struct Student *new_student) {
int size = sizeof(students) / sizeof(students[0]);
struct Student *temp = (struct Student *)realloc(students, (size + 1) * sizeof(struct Student));
if (temp != NULL) {
students = temp;
strcpy(students[size].id, new_student->id);
strcpy(students[size].name, new_student->name);
strcpy(students[size].gender, new_student->gender);
students[size].chinese = new_student->chinese;
students[size].math = new_student->math;
students[size].english = new_student->english;
printf("添加成功!\n");
} else {
printf("添加失败!\n");
}
}
```
这个函数首先计算出原数组中的元素个数,然后使用`realloc`函数动态分配更多的内存,使得数组的大小增加1。如果分配内存成功,就将新学生的信息复制到新的空间中,并输出添加成功的提示信息;否则输出添加失败的提示信息。注意,这里使用了`strcpy`函数来复制字符串类型的数据。
阅读全文
相关推荐
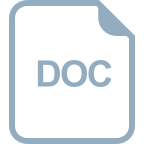
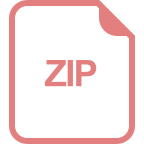
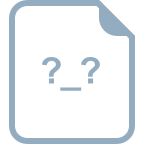















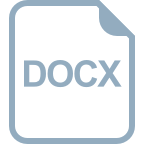