输入10个成绩,查找最高分并输出。 #include <stdio.h> int *GetMax(int score[ ], int n); int main(void) { int i, score[10], *p; for(i = 0; i < 10; i++){ scanf("%d", &score[i]); } p = *GetMax(score[i],n) ; printf("%d\n", *p); return 0; } int *GetMax(int score[ ], int n) { int i, max, pos = 0; max = score[0] ; for(i = 0 ; i < 10 ; i++){ if(score[i] > max){ max = score[i]; pos = i ; } } return max ; }
时间: 2024-01-28 15:05:32 浏览: 69
在这段代码中有一些问题:
1. 在 `main()` 函数中,调用 `GetMax()` 函数时,没有传入参数 `n`,导致编译时会出现错误。
2. 在 `GetMax()` 函数中,返回值类型是 `int*`,但是在函数体内使用的变量 `max` 的类型是 `int`,这也会导致编译时出现错误。
3. 在 `GetMax()` 函数中,返回值应该是指向最大值的指针,而不是最大值本身。
下面是修改后的代码:
```
#include <stdio.h>
int *GetMax(int score[], int n);
int main(void) {
int i, score[10], *p;
for(i = 0; i < 10; i++){
scanf("%d", &score[i]);
}
p = GetMax(score, 10);
printf("%d\n", *p);
return 0;
}
int *GetMax(int score[], int n) {
int i, max = score[0], pos = 0;
for(i = 1; i < n; i++){
if(score[i] > max){
max = score[i];
pos = i;
}
}
return &score[pos];
}
```
修改后的代码通过传入参数 `n` 来指定数组的长度,调用 `GetMax()` 函数时传入了正确的参数,同时在 `GetMax()` 函数中,使用指针保存最大值的地址,并返回该指针。
阅读全文
相关推荐
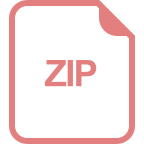
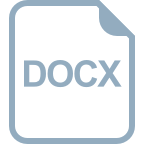
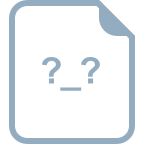















