#include <stdio.h> main() { FILE *fp; char str[10]; fp=fopen("myfile.dat","w"); fputs("abc",fp); fclose(fp); fp=fopen("myfile.dat","a+"); fprintf(fp,"%d",28); rewind(fp); fscanf(fp,"%s",str); puts(str); fclose(fp); }
时间: 2024-03-04 21:53:28 浏览: 261
这段代码是用C语言编写的,主要功能是向一个名为“myfile.dat”的文件中写入字符串“abc”,然后将文件指针移回文件开头,从文件中读取字符串并输出到屏幕。具体来说,代码中使用fopen()函数打开文件,使用fputs()函数将字符串写入文件中,再使用fclose()函数关闭文件。然后再次使用fopen()函数打开文件,使用fprintf()函数将数字28写入文件中,再使用rewind()函数将文件指针移回文件开头,使用fscanf()函数从文件中读取字符串,最后使用puts()函数将读取到的字符串输出到屏幕上。最后再使用fclose()函数关闭文件。
相关问题
现有一个已存放了用户加密信息的文件myfile.txt,该文件的生成就是把用户从键盘输入的字符与'x'进行异或运算,用户输入以'\n'结束。现在需要写一个程序,完成用户信息的加密并存放到myfile.txt中。 有两位同学想实现上述功能,分别完成了一个程序。 下列对两个程序的分析中正确的说法是( )。 //学生甲的程序 #include <stdio.h> #include <string.h> #include <stdlib.h> int main(void) { FILE *fp; int i; char ch; fp=fopen("myfile.txt","w+"); if(fp==NULL) { printf("File open error!\n"); exit(0); } ch=getchar(); while(ch!='\n') { ch=ch^'x'; fputc(ch,fp); ch=getchar(); } fclose(fp); return 0; } //学生乙的程序 #include <stdio.h> #include <string.h> #include <stdlib.h> int main(void) { FILE *fp; int i=0; char sec[100]; fp=fopen("myfile.txt","r+"); if(fp==NULL) { printf("File open error!\n"); exit(0); } gets(sec); while(sec[i]!='\0') { sec[i]=sec[i]^120; i++; } fputs(sec,fp); fclose(fp); return 0; }
学生甲的程序是将用户输入的字符与'x'进行异或运算后写入文件myfile.txt中,学生乙的程序是将已存在文件myfile.txt中的内容读入到char数组sec中,然后将数组sec中每个字符与120进行异或运算后再写回文件中。
由于学生乙的程序是以"r+"方式打开文件myfile.txt的,因此在写入文件时会覆盖原有的内容。而学生甲的程序是以"w+"方式打开文件myfile.txt的,每次写入文件都会截断文件并覆盖原有内容。因此,如果学生甲和学生乙的程序都运行,则最终文件myfile.txt中只会包含学生乙程序中加密后的内容。
因此,正确的说法是:学生乙的程序会覆盖学生甲程序写入的内容,最终文件中只包含学生乙程序中加密后的内容。
C语言程序填空:从键盘输入10个字符,将其全部输出到一个磁盘文件“data.dat"中保存起来#include<stdio.h> #include<conio.h> #include<stdlib.h> void main() { FILE *fp; int num; int i=0; if((fp=fopen("data.dat","w"))==NULL) { printf("打不开文件\n"); exit(0); } while(i<=9) { fprintf(fp,"%d",num); i++; } }
#include<stdio.h>
#include<conio.h>
#include<stdlib.h>
void main() {
FILE *fp;
char ch[10];
int i=0;
if((fp=fopen("data.dat","w"))==NULL) {
printf("打不开文件\n");
exit(0);
}
printf("请输入10个字符:\n");
while(i<=9) {
ch[i] = getch();
fprintf(fp,"%c",ch[i]);
i++;
}
fclose(fp);
printf("已将输入的字符保存到文件data.dat中!");
}
注意:程序中使用了 getch() 函数,需要包含 conio.h 头文件。另外,文件操作完成后需要关闭文件,使用 fclose() 函数。
阅读全文
相关推荐





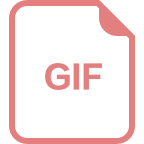










