android编辑购物车,Android制作简单的普通购物车代码
时间: 2023-06-26 21:08:55 浏览: 92
编辑购物车需要先设计好购物车的数据结构,通常包括商品名称、价格、数量、图片等信息。下面是一个简单的 Android 购物车代码示例:
1. 创建一个商品类
```
public class Product {
private String name;
private double price;
private int quantity;
private int image;
public Product(String name, double price, int quantity, int image) {
this.name = name;
this.price = price;
this.quantity = quantity;
this.image = image;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getQuantity() {
return quantity;
}
public int getImage() {
return image;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
```
2. 创建一个购物车类
```
public class ShoppingCart {
private List<Product> productList;
public ShoppingCart() {
productList = new ArrayList<>();
}
public void addProduct(Product product) {
productList.add(product);
}
public void removeProduct(Product product) {
productList.remove(product);
}
public List<Product> getProductList() {
return productList;
}
public double getTotalPrice() {
double totalPrice = 0;
for (Product product : productList) {
totalPrice += product.getPrice() * product.getQuantity();
}
return totalPrice;
}
}
```
3. 创建一个商品列表页面
```
public class ProductListActivity extends AppCompatActivity {
private List<Product> productList;
private RecyclerView recyclerView;
private ProductAdapter productAdapter;
private ShoppingCart shoppingCart;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_product_list);
// 初始化商品列表
productList = new ArrayList<>();
productList.add(new Product("商品1", 100, 0, R.drawable.product1));
productList.add(new Product("商品2", 200, 0, R.drawable.product2));
productList.add(new Product("商品3", 300, 0, R.drawable.product3));
// 初始化购物车
shoppingCart = new ShoppingCart();
// 初始化RecyclerView
recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
productAdapter = new ProductAdapter(productList, new ProductAdapter.OnItemClickListener() {
@Override
public void onItemClick(Product product) {
// 添加商品到购物车
shoppingCart.addProduct(product);
Toast.makeText(ProductListActivity.this, "已添加到购物车", Toast.LENGTH_SHORT).show();
}
});
recyclerView.setAdapter(productAdapter);
}
private class ProductHolder extends RecyclerView.ViewHolder {
private ImageView imageView;
private TextView nameTextView;
private TextView priceTextView;
public ProductHolder(LayoutInflater inflater, ViewGroup parent) {
super(inflater.inflate(R.layout.list_item_product, parent, false));
imageView = itemView.findViewById(R.id.product_image);
nameTextView = itemView.findViewById(R.id.product_name);
priceTextView = itemView.findViewById(R.id.product_price);
}
public void bind(Product product) {
imageView.setImageResource(product.getImage());
nameTextView.setText(product.getName());
priceTextView.setText(String.format(Locale.getDefault(), "¥%.2f", product.getPrice()));
}
}
private class ProductAdapter extends RecyclerView.Adapter<ProductHolder> {
private List<Product> productList;
private OnItemClickListener listener;
public ProductAdapter(List<Product> productList, OnItemClickListener listener) {
this.productList = productList;
this.listener = listener;
}
public interface OnItemClickListener {
void onItemClick(Product product);
}
@NonNull
@Override
public ProductHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
LayoutInflater inflater = LayoutInflater.from(ProductListActivity.this);
return new ProductHolder(inflater, parent);
}
@Override
public void onBindViewHolder(@NonNull ProductHolder holder, int position) {
Product product = productList.get(position);
holder.bind(product);
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onItemClick(product);
}
});
}
@Override
public int getItemCount() {
return productList.size();
}
}
}
```
4. 创建一个购物车页面
```
public class ShoppingCartActivity extends AppCompatActivity {
private RecyclerView recyclerView;
private ProductAdapter productAdapter;
private ShoppingCart shoppingCart;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_shopping_cart);
// 初始化购物车
shoppingCart = new ShoppingCart();
// 初始化RecyclerView
recyclerView = findViewById(R.id.recycler_view);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
productAdapter = new ProductAdapter(shoppingCart.getProductList(), new ProductAdapter.OnItemClickListener() {
@Override
public void onItemClick(Product product) {
// 从购物车中删除商品
shoppingCart.removeProduct(product);
productAdapter.notifyDataSetChanged();
}
});
recyclerView.setAdapter(productAdapter);
// 设置“结算”按钮的点击事件
Button checkoutButton = findViewById(R.id.checkout_button);
checkoutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(ShoppingCartActivity.this, "总价:" + shoppingCart.getTotalPrice(), Toast.LENGTH_SHORT).show();
}
});
}
private class ProductHolder extends RecyclerView.ViewHolder {
private ImageView imageView;
private TextView nameTextView;
private TextView priceTextView;
private TextView quantityTextView;
public ProductHolder(LayoutInflater inflater, ViewGroup parent) {
super(inflater.inflate(R.layout.list_item_shopping_cart, parent, false));
imageView = itemView.findViewById(R.id.product_image);
nameTextView = itemView.findViewById(R.id.product_name);
priceTextView = itemView.findViewById(R.id.product_price);
quantityTextView = itemView.findViewById(R.id.product_quantity);
}
public void bind(Product product) {
imageView.setImageResource(product.getImage());
nameTextView.setText(product.getName());
priceTextView.setText(String.format(Locale.getDefault(), "¥%.2f", product.getPrice()));
quantityTextView.setText(String.format(Locale.getDefault(), "x%d", product.getQuantity()));
}
}
private class ProductAdapter extends RecyclerView.Adapter<ProductHolder> {
private List<Product> productList;
private OnItemClickListener listener;
public ProductAdapter(List<Product> productList, OnItemClickListener listener) {
this.productList = productList;
this.listener = listener;
}
public interface OnItemClickListener {
void onItemClick(Product product);
}
@NonNull
@Override
public ProductHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
LayoutInflater inflater = LayoutInflater.from(ShoppingCartActivity.this);
return new ProductHolder(inflater, parent);
}
@Override
public void onBindViewHolder(@NonNull ProductHolder holder, int position) {
Product product = productList.get(position);
holder.bind(product);
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.onItemClick(product);
}
});
}
@Override
public int getItemCount() {
return productList.size();
}
}
}
```
以上代码是一个简单的购物车实现,包括商品列表页面和购物车页面。在商品列表页面点击商品,会将商品添加到购物车中,可以在购物车页面进行删除和结算操作。
阅读全文
相关推荐
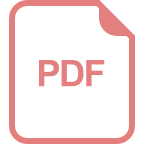

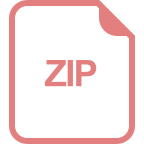
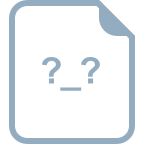


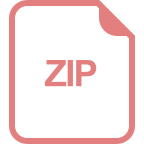







