Android 购物车代码
时间: 2023-11-03 11:53:59 浏览: 35
以下是一个简单的 Android 购物车示例代码,仅供参考:
首先是商品实体类:
```java
public class Product {
private String name;
private double price;
private int quantity;
public Product(String name, double price, int quantity) {
this.name = name;
this.price = price;
this.quantity = quantity;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
}
```
接下来是购物车管理类:
```java
public class CartManager {
private List<Product> cart = new ArrayList<>();
public List<Product> getCart() {
return cart;
}
public void addProductToCart(Product product) {
for (Product p : cart) {
if (p.getName().equals(product.getName())) {
p.setQuantity(p.getQuantity() + 1);
return;
}
}
cart.add(product);
}
public void removeProductFromCart(Product product) {
for (Product p : cart) {
if (p.getName().equals(product.getName())) {
if (p.getQuantity() > 1) {
p.setQuantity(p.getQuantity() - 1);
} else {
cart.remove(p);
}
break;
}
}
}
public double getTotalPrice() {
double totalPrice = 0.0;
for (Product p : cart) {
totalPrice += p.getPrice() * p.getQuantity();
}
return totalPrice;
}
}
```
在 Activity 中使用购物车管理类:
```java
public class MainActivity extends AppCompatActivity {
private CartManager cartManager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
cartManager = new CartManager();
// 添加商品到购物车
Product product1 = new Product("商品1", 100.0, 1);
cartManager.addProductToCart(product1);
Product product2 = new Product("商品2", 200.0, 1);
cartManager.addProductToCart(product2);
// 从购物车中移除商品
cartManager.removeProductFromCart(product1);
// 获取购物车中的商品列表和总价
List<Product> cart = cartManager.getCart();
double totalPrice = cartManager.getTotalPrice();
}
}
```
以上仅为一个简单的示例,实际开发中需要根据具体的业务需求进行开发。
相关推荐
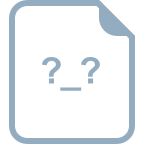













