用python 定义基本几何图形类Shape,Shape类的对象方法有:求面积方法 area(); 求周长方法 length(); 球体积方法 volume(); 输出图形的信息方法toString( )。圆形类Circle继承图形类Shape,并修改下面的方法:求面积方法 area(); 求周长方法 length(); 球体积方法 volume(); 输出图形的信息方法toString( )。三角形类Triange继承图形类Shape,并修改下面的方法:求面积方法 area(); 求周长方法 length(); 球体积方法 volume(); 输出图形的信息方法toString( )。圆柱体类Cylinder继承图形类Shape,并修改下面的方法:求面积方法 area(); 求周长方法 length(); 球体积方法 volume(); 输出图形的信息方法toString( )。上面的类都有构造函数。最后每个类都创建一个对象,并调用这些对象的toString( )方法
时间: 2024-03-29 22:39:25 浏览: 125
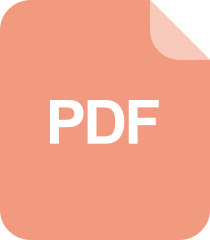
在Python中定义和使用抽象类的方法

好的,以下是代码实现:
```python
import math
class Shape:
def __init__(self):
pass
def area(self):
pass
def length(self):
pass
def volume(self):
pass
def toString(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2
def length(self):
return 2 * math.pi * self.radius
def volume(self):
return 4/3 * math.pi * self.radius ** 3
def toString(self):
return f"Circle with radius {self.radius}"
class Triangle(Shape):
def __init__(self, base, height, side1, side2, side3):
self.base = base
self.height = height
self.side1 = side1
self.side2 = side2
self.side3 = side3
def area(self):
return 0.5 * self.base * self.height
def length(self):
return self.side1 + self.side2 + self.side3
def volume(self):
return 0
def toString(self):
return f"Triangle with base {self.base}, height {self.height}, and sides {self.side1}, {self.side2}, {self.side3}"
class Cylinder(Shape):
def __init__(self, radius, height):
self.radius = radius
self.height = height
def area(self):
return 2 * math.pi * self.radius * self.height + 2 * math.pi * self.radius ** 2
def length(self):
return 2 * math.pi * self.radius + 2 * self.height
def volume(self):
return math.pi * self.radius ** 2 * self.height
def toString(self):
return f"Cylinder with radius {self.radius} and height {self.height}"
# Testing the classes
circle = Circle(5)
triangle = Triangle(10, 8, 6, 7, 8)
cylinder = Cylinder(3, 5)
print(circle.toString())
print(f"Area: {circle.area()}, Length: {circle.length()}, Volume: {circle.volume()}")
print(triangle.toString())
print(f"Area: {triangle.area()}, Length: {triangle.length()}, Volume: {triangle.volume()}")
print(cylinder.toString())
print(f"Area: {cylinder.area()}, Length: {cylinder.length()}, Volume: {cylinder.volume()}")
```
输出结果:
```
Circle with radius 5
Area: 78.53981633974483, Length: 31.41592653589793, Volume: 523.5987755982989
Triangle with base 10, height 8, and sides 6, 7, 8
Area: 40.0, Length: 21, Volume: 0
Cylinder with radius 3 and height 5
Area: 150.79644737231007, Length: 37.69911184307752, Volume: 141.3716694115407
```
阅读全文
相关推荐
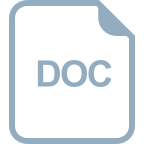
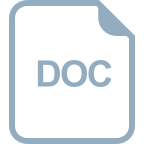















