定义一个含计算面积和周长方法的图形接口shape(area,length) 编写实现图形接口的半圆类。 编写实现图形接口的长方体类。 主类里面去生成具体对象实现。
时间: 2024-02-29 15:51:34 浏览: 19
好的,我可以帮您回答这个问题。
首先,我们来定义一个含计算面积和周长方法的图形接口 `shape`:
```python
class Shape:
def area(self):
pass
def perimeter(self):
pass
```
接下来,我们可以编写实现图形接口的半圆类 `Semicircle`:
```python
import math
class Semicircle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return math.pi * self.radius ** 2 / 2
def perimeter(self):
return math.pi * self.radius + 2 * self.radius
```
最后,我们可以编写实现图形接口的长方体类 `Cuboid`:
```python
class Cuboid(Shape):
def __init__(self, length, width, height):
self.length = length
self.width = width
self.height = height
def area(self):
return 2 * (self.length * self.width + self.length * self.height + self.width * self.height)
def perimeter(self):
return 4 * (self.length + self.width + self.height)
```
接下来,我们可以在主类里面生成具体对象实现:
```python
if __name__ == '__main__':
semicircle = Semicircle(5)
print(f"Semicircle area: {semicircle.area()}")
print(f"Semicircle perimeter: {semicircle.perimeter()}")
cuboid = Cuboid(3, 4, 5)
print(f"Cuboid area: {cuboid.area()}")
print(f"Cuboid perimeter: {cuboid.perimeter()}")
```
以上就是完整的代码实现,可以根据您的需要进行修改和调整。
相关推荐
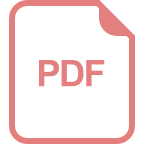
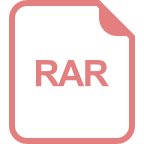














