verilog AXI slave with outstanding
时间: 2023-08-24 16:04:59 浏览: 145
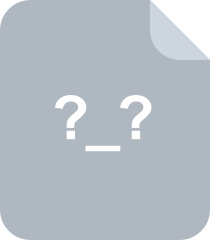
verilog highlight
An AXI slave with outstanding transactions can be implemented in Verilog by using a state machine to manage the response to incoming transactions. The state machine can keep track of the number of outstanding transactions and respond accordingly. Here is an example implementation:
```
module axi_slave_outstanding(
input aclk,
input aresetn,
input awvalid,
input [31:0] awaddr,
input [2:0] awprot,
input awcache,
input awburst,
input [3:0] awlen,
input arvalid,
input [31:0] araddr,
input [2:0] arprot,
input arcache,
input arburst,
input [3:0] arlen,
input wvalid,
input [31:0] wdata,
input [3:0] wstrb,
input wlast,
output bvalid,
output rvalid,
output [1:0] rresp,
output [31:0] rdata,
output rlast
);
reg [1:0] state;
reg [31:0] mem[0:1023];
reg [3:0] arcount;
reg [3:0] awcount;
// Reset state machine and counters on reset
always @(posedge aclk) begin
if (!aresetn) begin
state <= 2'b00;
arcount <= 4'b0000;
awcount <= 4'b0000;
end
end
// State machine
always @(posedge aclk) begin
case (state)
// Idle state
2'b00: begin
if (awvalid) begin
state <= 2'b01;
awcount <= awlen;
end else if (arvalid) begin
state <= 2'b10;
arcount <= arlen;
end
end
// Write data state
2'b01: begin
if (wvalid) begin
mem[awaddr] <= wdata;
awaddr <= awaddr + 1;
awcount <= awcount - 1;
if (wlast) begin
bvalid <= 1'b1;
state <= 2'b00;
end
end
end
// Read data state
2'b10: begin
if (rcount == 4'b0000) begin
rvalid <= 1'b1;
rdata <= mem[araddr];
end
araddr <= araddr + 1;
arcount <= arcount - 1;
if (arcount == 4'b0000) begin
rlast <= 1'b1;
rvalid <= 1'b0;
state <= 2'b00;
end
end
// Error state
default: begin
state <= 2'b00;
end
endcase
end
endmodule
```
In this implementation, the state machine has three states: idle, write data, and read data. When an AXI write transaction is received, the state machine transitions to the write data state and writes the incoming data to memory. It also keeps track of the number of outstanding write transactions using the `awcount` counter. When an AXI read transaction is received, the state machine transitions to the read data state and reads the requested data from memory. It also keeps track of the number of outstanding read transactions using the `arcount` counter.
The `bvalid`, `rvalid`, `rdata`, and `rlast` outputs are used to signal the completion of the AXI transactions to the master. The `bvalid` output is set to 1 when a write response is ready, and the `rvalid` output is set to 1 when a read response is ready. The `rdata` output contains the read data, and the `rlast` output signals the end of a read transaction.
This implementation assumes a single master and a single slave, and does not support burst transactions or interleaved transactions. It also does not support any AXI extensions beyond the basic read and write transactions.
阅读全文
相关推荐
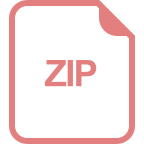
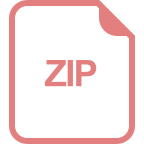
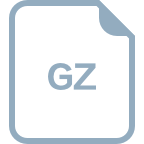
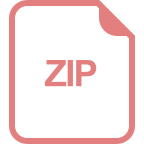
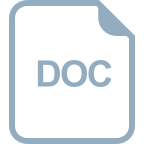

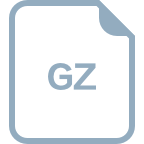
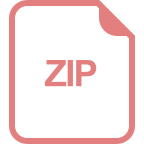
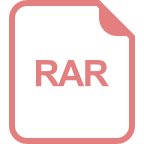
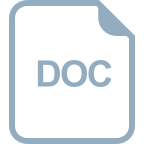





