WPF 中向Canvas动态添加控件时,如果Canvas对应位置上已有控件,怎么让动态添加的控件换个地方添加
时间: 2024-03-04 14:52:43 浏览: 157
在向Canvas动态添加控件时,可以使用Canvas.SetLeft和Canvas.SetTop方法设置控件的位置。如果对应位置已有控件,可以通过更改这些属性的值来将控件添加到新的位置。可以使用Canvas.GetLeft和Canvas.GetTop方法获取已有控件的位置信息,然后根据需要进行调整。同时,还可以使用Canvas.Children属性获取Canvas中已有的所有控件,以便进行位置比较和调整。
相关问题
WPF 中向Canvas动态添加控件时,如果Canvas对应位置上已有控件,怎么让动态添加的控件换个地方添加?提供具体实现代码
可以使用Canvas.SetLeft和Canvas.SetTop方法来设置控件在Canvas中的位置。如果要动态添加的控件的位置与Canvas中已有的控件重叠,可以通过逐步增加控件的左偏移和上偏移来避免位置重叠。以下是一个示例代码:
```csharp
// 创建一个新的控件
var newControl = new Button();
newControl.Content = "New Control";
// 设置控件的初始位置
Canvas.SetLeft(newControl, 100);
Canvas.SetTop(newControl, 100);
// 检查控件位置是否与Canvas中已有的控件重叠
while (Canvas.GetLeft(newControl) + newControl.Width > canvas.ActualWidth ||
Canvas.GetTop(newControl) + newControl.Height > canvas.ActualHeight ||
CheckOverlap(newControl, canvas.Children))
{
// 增加左偏移和上偏移,避免位置重叠
Canvas.SetLeft(newControl, Canvas.GetLeft(newControl) + 10);
Canvas.SetTop(newControl, Canvas.GetTop(newControl) + 10);
}
// 将控件添加到Canvas中
canvas.Children.Add(newControl);
// 检查控件是否与其他控件重叠
private bool CheckOverlap(UIElement element, UIElementCollection collection)
{
Rect elementRect = new Rect(Canvas.GetLeft(element), Canvas.GetTop(element), element.RenderSize.Width, element.RenderSize.Height);
foreach (UIElement child in collection)
{
Rect childRect = new Rect(Canvas.GetLeft(child), Canvas.GetTop(child), child.RenderSize.Width, child.RenderSize.Height);
if (elementRect.IntersectsWith(childRect))
{
return true;
}
}
return false;
}
```
这段代码可以在一个Canvas中动态添加控件,并且避免控件位置重叠。在这个示例中,我们首先创建一个新的控件,然后设置它的初始位置。接着,我们使用一个while循环来检查控件位置是否与Canvas中已有的控件重叠。如果重叠了,我们就逐步增加控件的左偏移和上偏移,直到避免位置重叠为止。最后,我们将控件添加到Canvas中。
WPF 中向Canvas动态添加控件,该控件要求在被点击的时候有虚线方框包裹控件,用鼠标拖动边框的四条边可以改变边框大小且动态改变控件的大小,但是边框及边框内控件在Canvas上的定位不发生变化。点击Canvas空白处时,取消掉包裹控件的边框
你可以实现一个自定义的控件类,继承自Canvas,然后在该类中实现鼠标事件的处理。在该类中,你需要维护一个当前被包裹的控件的引用,以及该控件的边框。
在鼠标点击事件中,你需要判断当前鼠标点击的位置是否在某个子控件上,如果是,则将该子控件作为当前被包裹的控件,并创建边框并添加到Canvas上。在鼠标移动事件中,你需要判断当前是否在拖动边框,如果是,则改变边框大小,并动态调整当前被包裹的控件的大小。在鼠标释放事件中,你需要将当前被包裹的控件的引用和边框清空。
以下是一个简单的示例代码,可以实现你的需求:
```csharp
public class ResizableCanvas : Canvas
{
private FrameworkElement _selectedElement;
private Rectangle _selectionBorder;
public ResizableCanvas()
{
this.MouseLeftButtonDown += OnMouseLeftButtonDown;
this.MouseLeftButtonUp += OnMouseLeftButtonUp;
this.MouseMove += OnMouseMove;
this.MouseLeave += OnMouseLeave;
}
private void OnMouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
// 判断是否在子控件上点击
var element = e.Source as FrameworkElement;
if (element != null)
{
_selectedElement = element;
_selectionBorder = new Rectangle
{
Stroke = Brushes.Black,
StrokeDashArray = new DoubleCollection(new double[] { 4, 4 }),
StrokeThickness = 1,
Fill = Brushes.Transparent
};
this.Children.Add(_selectionBorder);
Canvas.SetLeft(_selectionBorder, Canvas.GetLeft(_selectedElement));
Canvas.SetTop(_selectionBorder, Canvas.GetTop(_selectedElement));
_selectionBorder.Width = _selectedElement.ActualWidth;
_selectionBorder.Height = _selectedElement.ActualHeight;
}
}
private void OnMouseMove(object sender, MouseEventArgs e)
{
// 判断是否在拖动边框
if (_selectedElement != null && _selectionBorder != null)
{
var pos = e.GetPosition(this);
var dx = pos.X - Canvas.GetLeft(_selectedElement);
var dy = pos.Y - Canvas.GetTop(_selectedElement);
var newWidth = Math.Max(dx, 0);
var newHeight = Math.Max(dy, 0);
_selectionBorder.Width = newWidth;
_selectionBorder.Height = newHeight;
_selectedElement.Width = newWidth;
_selectedElement.Height = newHeight;
}
}
private void OnMouseLeftButtonUp(object sender, MouseButtonEventArgs e)
{
// 清空选中状态
_selectedElement = null;
if (_selectionBorder != null)
{
this.Children.Remove(_selectionBorder);
_selectionBorder = null;
}
}
private void OnMouseLeave(object sender, MouseEventArgs e)
{
// 取消选中状态
_selectedElement = null;
if (_selectionBorder != null)
{
this.Children.Remove(_selectionBorder);
_selectionBorder = null;
}
}
}
```
在使用该控件时,你可以像使用普通的Canvas一样添加子控件,例如:
```xml
<local:ResizableCanvas>
<Button Content="Button 1" Width="100" Height="50" Canvas.Left="50" Canvas.Top="50"/>
<Button Content="Button 2" Width="100" Height="50" Canvas.Left="150" Canvas.Top="150"/>
</local:ResizableCanvas>
```
阅读全文
相关推荐
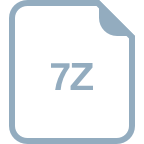
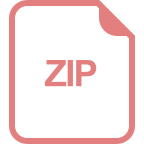
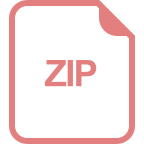












