python 去掉\t \n
时间: 2023-07-12 09:54:05 浏览: 45
你可以使用字符串的`strip()`方法来去掉字符串两端的空白字符,包括`\t`和`\n`。如果你想去掉字符串中间的空白字符,可以使用字符串的`replace()`方法来替换。例如:
```python
s = '\t hello \n world \t'
s = s.strip() # 去掉两端空白字符
s = s.replace('\t', '').replace('\n', '') # 去掉中间的空白字符
print(s) # 输出:hello world
```
另外,还可以使用正则表达式来去掉空白字符。例如:
```python
import re
s = '\t hello \n world \t'
s = re.sub(r'\s+', '', s) # 去掉所有空白字符
print(s) # 输出:helloworld
```
相关问题
python 如何去除\n\t
在Python中,可以使用字符串的`replace()`方法来去除`\n`和`\t`。`replace()`方法接受两个参数,第一个参数是要替换的子字符串,第二个参数是替换后的字符串。下面是一个示例:
```python
# 原始字符串
text = "Hello\n\tWorld!"
# 使用replace()方法去除\n和\t
clean_text = text.replace("\n", "").replace("\t", "")
print(clean_text)
```
输出结果为:
```
HelloWorld!
```
python去除json中\t\r\n
您可以使用`json.loads()`方法将JSON字符串解析为Python对象,然后再使用`json.dumps()`方法将Python对象转换回JSON字符串。在转换过程中,可以使用`replace()`方法去除`\t`、`\r`和`\n`等特殊字符。
以下是一个示例代码:
```python
import json
json_str = '{"key1": "value1\t", "key2": "value2\r\n"}'
# 解析JSON字符串为Python对象
data = json.loads(json_str)
# 将Python对象转换为JSON字符串,并去除特殊字符
clean_json_str = json.dumps(data, ensure_ascii=False).replace('\t', '').replace('\r', '').replace('\n', '')
print(clean_json_str)
```
输出结果:
```
{"key1": "value1", "key2": "value2"}
```
在这个示例中,我们使用`replace()`方法去除了`\t`、`\r`和`\n`字符,并通过将`ensure_ascii`设置为`False`来确保非ASCII字符正常显示。
相关推荐
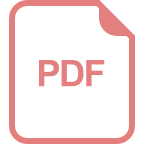
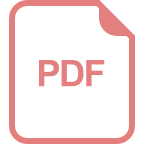
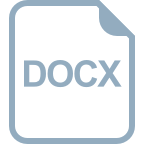
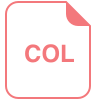
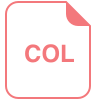
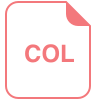
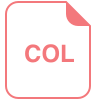
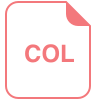







