windows和linux下面如何区分导出函数的关键字
时间: 2024-02-22 21:55:12 浏览: 19
在Windows下,函数导出需要使用__declspec(dllexport)关键字进行标识,如下所示:
```cpp
__declspec(dllexport) int add(int a, int b)
{
return a + b;
}
```
在Linux下,函数导出需要使用__attribute__((visibility("default")))关键字进行标识,如下所示:
```cpp
__attribute__((visibility("default"))) int add(int a, int b)
{
return a + b;
}
```
需要注意的是,在Linux下,如果不使用__attribute__((visibility("default")))关键字进行标识,函数默认是不导出的,而在Windows下,如果不使用__declspec(dllexport)关键字进行标识,函数默认是不导出的。
相关问题
linux下用什么关键字定义导出函数和变量的属性
在Linux下,可以使用关键字`__attribute__((visibility("default")))`来定义导出函数和变量的属性。这个关键字告诉编译器将这些函数和变量作为可见的符号导出,以便在链接时可以被其他程序或库使用。在程序中使用这个关键字的语法如下:
```
__attribute__((visibility("default"))) int my_variable = 42;
__attribute__((visibility("default"))) void my_function() {
// function body
}
```
其中,`my_variable`和`my_function`都会被作为可见符号导出。
如何在windows和linux上区分链接 pthread
在 Windows 上,使用 POSIX Threads for Windows(pthreads-win32)库时,需要链接 `-lpthread`。在 Linux 上,也需要链接 `-lpthread`。
可以使用以下命令进行链接:
在 Windows 上:
```
gcc example.c -o example.exe -lpthread
```
在 Linux 上:
```
gcc example.c -o example -lpthread
```
注意,在 Windows 上需要先安装 pthreads-win32 库,才能使用 `-lpthread` 进行链接。
相关推荐
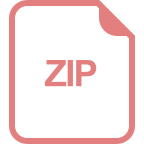
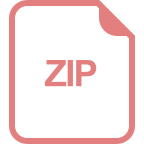
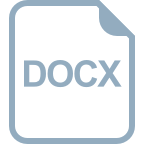












