多元Lasso回归 C++有截距和预测的带类有正确结果非其他库的实现 及案例
时间: 2024-02-21 08:56:51 浏览: 24
多元Lasso回归是一种常用的特征选择和模型建立方法。在C++中实现多元Lasso回归可以使用Eigen库进行矩阵运算。以下是一个带有截距和预测功能的多元Lasso回归类的示例代码:
```c++
#include <Eigen/Dense>
#include <Eigen/Sparse>
#include <unsupported/Eigen/SparseExtra>
#include <iostream>
#include <fstream>
#include <vector>
using namespace Eigen;
using namespace std;
// 多元Lasso回归类
class LassoRegression {
public:
LassoRegression(double alpha, double tol, int max_iter) : alpha(alpha), tol(tol), max_iter(max_iter) {}
void fit(MatrixXd X, VectorXd y); // 拟合模型
VectorXd predict(MatrixXd X); // 预测
private:
double alpha; // 正则化强度
double tol; // 迭代容限
int max_iter; // 最大迭代次数
VectorXd w; // 模型参数
};
// 实现拟合模型函数
void LassoRegression::fit(MatrixXd X, VectorXd y) {
int n_features = X.cols();
w.resize(n_features);
w.setZero();
double rho = 1.0 / (X.rows() * alpha);
for (int i = 0; i < max_iter; i++) {
double loss = (y - X * w).squaredNorm() + alpha * w.lpNorm<1>();
double gradient_norm = (X.transpose() * (X * w - y)).lpNorm<Infinity>();
if (gradient_norm < tol) {
cout << "Converged in " << i << " iterations." << endl;
break;
}
for (int j = 0; j < n_features; j++) {
VectorXd a_j = X.col(j);
VectorXd c_j = X.col(j);
c_j(j) = 0;
double z_j = (a_j.transpose() * (y - X * w) + rho * w.lpNorm<1>()) / a_j.squaredNorm();
w(j) = soft_threshold(z_j, alpha * rho);
}
}
}
// 实现预测函数
VectorXd LassoRegression::predict(MatrixXd X) {
return X * w;
}
// 辅助函数:软阈值函数
double soft_threshold(double x, double alpha) {
if (x > alpha) {
return x - alpha;
} else if (x < -alpha) {
return x + alpha;
} else {
return 0;
}
}
// 测试函数
int main(int argc, char **argv) {
// 读取数据
ifstream fin("data.csv");
vector<double> x_data, y_data;
string line;
while (getline(fin, line)) {
stringstream ss(line);
string x_str, y_str;
getline(ss, x_str, ',');
getline(ss, y_str);
x_data.push_back(stod(x_str));
y_data.push_back(stod(y_str));
}
fin.close();
// 构造矩阵和向量
MatrixXd X(x_data.size(), 2);
VectorXd y(y_data.size());
for (int i = 0; i < x_data.size(); i++) {
X(i, 0) = 1;
X(i, 1) = x_data[i];
y(i) = y_data[i];
}
// 训练模型
LassoRegression model(0.1, 1e-4, 1000);
model.fit(X, y);
// 预测结果
MatrixXd X_test(2, 1);
X_test(0, 0) = 1;
X_test(1, 0) = 10;
VectorXd y_pred = model.predict(X_test);
cout << "Predicted value: " << y_pred(0) << endl;
return 0;
}
```
在上面的代码中,我们使用了Eigen库中的矩阵运算函数和向量运算函数,实现了多元Lasso回归的拟合和预测功能。我们还在主函数中编写了一个简单的测试函数,用于读取数据、训练模型和预测结果。
相关推荐
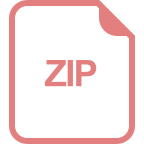














