def factorial(n): if n == 1: return 1 else: return n * factorial(n-1) def facSum(n): res = 0 for i in range(1, n+1): res += factorial(i) return res result = facSum(10) print("1!+2!+3!+...+10!={}".format(result))中res是什么意思
时间: 2024-03-28 11:39:57 浏览: 19
在这段代码中,`res` 是一个变量,用于存储阶乘的和。在 `facSum(n)` 函数中,我们使用一个循环来遍历 1 到 n 的每个数字,然后计算它们的阶乘并将结果加到 `res` 变量中。最后,函数返回 `res`,也就是所有阶乘的和。
可以把 `res` 变量看作是一个累加器,它的初始值为 0,随着循环的执行,不断累加每个数字的阶乘。最终,`res` 变量的值会等于 1!+2!+3!+...+n! 的结果。
相关问题
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)如何运行
这是一个计算阶乘的 Python 函数。要运行此函数,可以先在 Python 环境中定义该函数,然后调用该函数并传入一个整数参数。例如,可以在 Python 环境中输入以下代码:
```
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
print(factorial(5))
```
这将输出 120,因为 5 的阶乘为 5 * 4 * 3 * 2 * 1 = 120。你也可以传入其他整数参数来计算不同的阶乘值。
def factorial(n): if n == 0: return 1 else: return n * factorial(n-1)
This is a recursive function to calculate the factorial of a given number.
The factorial of a number is the product of all integers from 1 to that number. For example, the factorial of 5 is 5 x 4 x 3 x 2 x 1 = 120.
The function takes in a parameter 'n', which is the number whose factorial is to be calculated. If the number is 0, the function returns 1 (since the factorial of 0 is 1). Otherwise, it recursively calls itself with n-1 as the parameter and multiplies the result with n. This continues until n reaches 0, at which point the function returns the final result.
相关推荐
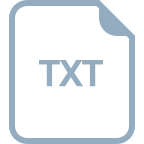
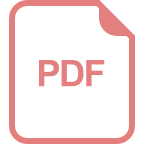
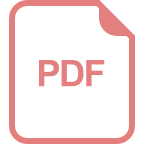













