用java编写一个智能家居
时间: 2023-08-19 13:05:44 浏览: 41
智能家居是指利用计算机、网络通信技术以及各种传感器和执行器等设备,对家庭进行智能化控制和管理。下面是一个简单的智能家居的Java实现。
1. 确定需求
首先需要明确智能家居的功能需求,比如:
- 控制家电开关状态
- 监测环境参数(如温度、湿度等)
- 触发报警或警告
- 远程控制
2. 设计架构
在确定了需求后,需要设计系统架构。智能家居的系统通常包括以下几个部分:
- 感知层:负责采集环境参数和控制家电开关状态
- 通信层:负责与用户终端或远程服务器进行通信
- 控制层:负责处理用户指令、自动控制等功能
3. 编写代码
在设计好系统架构后,就可以开始编写代码了。以下是一个简单的智能家居的Java实现示例:
```java
class SmartHome {
private boolean airCondStatus = false; // 空调状态
private boolean lightStatus = false; // 灯光状态
private int temperature = 20; // 温度,初始值为20度
public void setAirCondStatus(boolean status) {
airCondStatus = status;
System.out.println("空调状态已设置为:" + status);
}
public void setLightStatus(boolean status) {
lightStatus = status;
System.out.println("灯光状态已设置为:" + status);
}
public int getTemperature() {
return temperature;
}
public void setTemperature(int temperature) {
this.temperature = temperature;
System.out.println("温度已设置为:" + temperature);
}
}
class Sensor {
private SmartHome smartHome;
public Sensor(SmartHome smartHome) {
this.smartHome = smartHome;
}
public void detectTemperature() {
int temperature = ... // 从传感器读取温度数据
smartHome.setTemperature(temperature);
}
}
class Actuator {
private SmartHome smartHome;
public Actuator(SmartHome smartHome) {
this.smartHome = smartHome;
}
public void turnOnAirCond() {
smartHome.setAirCondStatus(true);
}
public void turnOffAirCond() {
smartHome.setAirCondStatus(false);
}
public void turnOnLight() {
smartHome.setLightStatus(true);
}
public void turnOffLight() {
smartHome.setLightStatus(false);
}
}
class UserInterface {
private SmartHome smartHome;
public UserInterface(SmartHome smartHome) {
this.smartHome = smartHome;
}
public void controlAirCond(boolean status) {
Actuator actuator = new Actuator(smartHome);
if (status) {
actuator.turnOnAirCond();
} else {
actuator.turnOffAirCond();
}
}
public void controlLight(boolean status) {
Actuator actuator = new Actuator(smartHome);
if (status) {
actuator.turnOnLight();
} else {
actuator.turnOffLight();
}
}
public void displayTemperature() {
int temperature = smartHome.getTemperature();
System.out.println("当前温度为:" + temperature);
}
}
public class Main {
public static void main(String[] args) {
SmartHome smartHome = new SmartHome();
Sensor sensor = new Sensor(smartHome);
UserInterface userInterface = new UserInterface(smartHome);
// 周期性地检测温度
while (true) {
sensor.detectTemperature();
userInterface.displayTemperature();
try {
Thread.sleep(1000); // 间隔1秒钟读取一次温度
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
以上代码实现了一个简单的智能家居,包括温度采集、空调和灯光控制等功能。可以根据自己的需求进行修改和扩展。
相关推荐
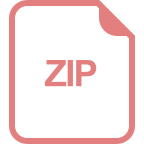
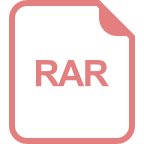
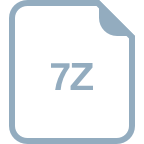
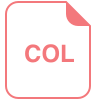
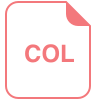
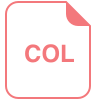
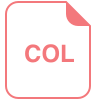
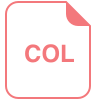









