ValueError: The output file must be a pkl file.
时间: 2024-02-26 08:57:16 浏览: 110
这个错误通常是由于输出文件的格式不正确导致的。你需要确保你的输出文件是一个 pkl 文件,而不是其他格式的文件,比如 txt、csv 等。如果你使用的是 Python 的 pickle 库,可以使用以下代码将输出保存为 pkl 文件:
```python
import pickle
# your code to generate output
with open('output.pkl', 'wb') as f:
pickle.dump(output, f)
```
这将把你的输出保存到名为 output.pkl 的文件中。你可以根据自己的需要修改文件名和路径。
相关问题
ValueError: x and y must be the same size
This error message usually occurs when you are trying to plot two arrays of different sizes against each other.
To fix this error, you need to ensure that the x and y arrays have the same number of elements. You could also check if there are any missing or NaN values in either of the arrays that may be causing the problem.
If you are using a plotting library like Matplotlib, you can check the size of your arrays using the `len()` function. For example:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40] # This will raise a ValueError
print(len(x)) # Output: 5
print(len(y)) # Output: 4
plt.plot(x, y)
plt.show()
```
In this example, the `y` array has one less value than the `x` array, causing the `ValueError`. You could fix this error by making both arrays the same size, for example by adding a missing value to `y`:
```
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [10, 20, 30, 40, None] # Add a missing value to y
print(len(x)) # Output: 5
print(len(y)) # Output: 5
plt.plot(x, y)
plt.show()
```
ValueError: Series.dtypes must be int, float or bool
这个错误通常出现在使用 pandas 库时,当你想对一个 Series 进行某些数值计算或者统计分析时,数据类型必须是 int、float 或 bool 类型。如果你的 Series 存在其他类型的数据,就会抛出这个错误。
为了解决这个问题,你可以尝试以下几个步骤:
1. 确认你的 Series 中是否包含了非 int、float 或 bool 类型的数据,比如字符串、日期等。可以使用 .dtype 属性来查看 Series 的数据类型。
2. 如果确实存在其他类型的数据,你可以尝试使用 .astype() 方法将其转换成 int、float 或 bool 类型。
3. 如果你不需要这些非数值类型的数据,可以使用 .drop() 方法将其删除。
以下是一个例子:
```
import pandas as pd
# 创建一个包含不同数据类型的 Series
s = pd.Series([1, 2.0, '3', True])
# 抛出错误
s.mean()
# 转换数据类型
s = s.astype(float)
# 计算平均值
s.mean()
```
相关推荐
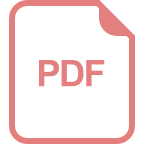
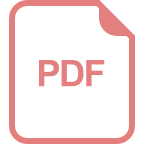
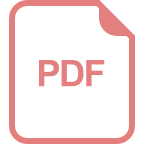












