linux c socketcan,写一个can0接口,使用canfd_frame的recvfrome函数接收程序和sendto
时间: 2024-03-17 17:41:55 浏览: 29
函数发送数据的示例代码可以是这样的:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main()
{
int sockfd;
struct sockaddr_can addr;
struct ifreq ifr;
struct canfd_frame frame;
// 创建socket
sockfd = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if (sockfd < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
// 设置can0接口
strcpy(ifr.ifr_name, "can0");
ioctl(sockfd, SIOCGIFINDEX, &ifr);
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(sockfd, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
exit(EXIT_FAILURE);
}
// 接收数据
while (1) {
ssize_t len = recvfrom(sockfd, &frame, sizeof(frame), 0, NULL, NULL);
if (len < 0) {
perror("recvfrom");
exit(EXIT_FAILURE);
}
printf("Received CAN frame with ID %d and DLC %d\n",
frame.can_id, frame.len);
for (int i = 0; i < frame.len; i++) {
printf("%02X ", frame.data[i]);
}
printf("\n");
}
// 发送数据
frame.can_id = 0x123;
frame.len = 8;
for (int i = 0; i < frame.len; i++) {
frame.data[i] = i;
}
if (sendto(sockfd, &frame, sizeof(frame), 0, (struct sockaddr *)&addr,
sizeof(addr)) < 0) {
perror("sendto");
exit(EXIT_FAILURE);
}
close(sockfd);
return 0;
}
```
这个程序首先创建了一个socket,然后绑定到了can0接口上。之后进入一个循环,不断地接收CAN数据帧。当接收到一个数据帧时,程序会打印出CAN帧的ID和DLC,并且把CAN帧的数据打印出来。最后,程序发送了一个CAN数据帧,它的CAN ID是0x123,数据长度是8,数据部分是0~7的连续数字。
注意:这个程序只是示例代码,没有处理CAN帧的错误情况,也没有检查发送数据的返回值。在实际应用中,需要根据具体情况进行修改和完善。
相关推荐
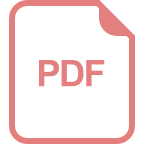
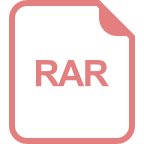
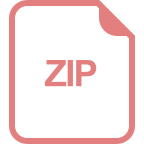














